The old DataFileHost website was a free file hosting site that allowed users to upload and share files with friends and family over the internet. However, for some reason, the previous owner had shut down the website and had put up the domain name for auction.
The reason for shutting down the website is not known.
We received a large number of emails from the old DataFileHost users that wanted access to the files that they had uploaded. So we thought to publish this article to those who are not aware of what had happened.
About the new DataFileHost
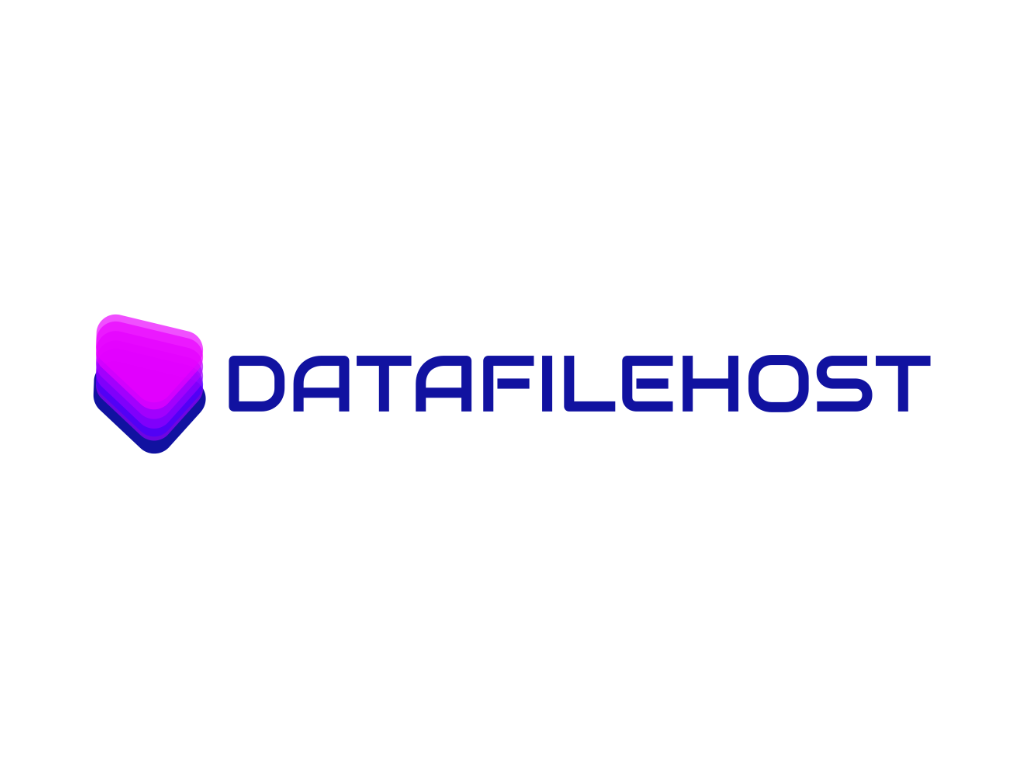
The domain is now managed by us (the new owners), that have no affiliation with the previous owner or their file hosting service.
Upon taking over the domain, we decided to run DataFileHost.com as a general news blog.
Posts
Business
- Decoding Posture: Enhancing Spinal Alignment with Posture Bra
- Which Broadband is Best for Business?
- Best Blockchain to Create a Crypto Token
- Boulevard Coast Jalan Loyang Besar EC A Hub of Convenience and Vibrancy for Residents with a Variety of Retail and Recreational Options
- How to Choose the Best Law Firm
- What Are the Eligibility Criteria for Low-Interest Personal Loans?
- 4 Reasons Why You Should Hire Digital Nomads
- Best Paid Survey Site to Make Extra Money
- 10 Awesome Video Types to Promote Your Business
- 10 Books To Scale Up And Build A Better Company
- 10 Unique Dropshipping Products to Sell in 2022
- 11 Packaging & Label Design ideas Help your Business Growth
- 2023 Forecast – What’s in Store for EUR/USD?
- 4 Advantages of Buying Bitcoin Now
- 4 Devastating Ways Blocked Gutters Can Damage Your Home
- 4 Ideas to Spice Up Your Marketing Strategy
- 4 Obvious Benefits of Professional Carpet Cleaning
- 4 Reasons Why You Need High-Performance Inserts in Metalworking
- 4 Tech Tips to Find the Best Condominium Online
- 4 Tips for Managing the Online Reputation of Your Small Business
- 4 Tips to Build Your Demand Gen Marketing Strategy for 2022
- 4 Tips to Choose the Best Location For Your Next Home
- 4 Tips to Land a Role in the DevOps Industry
- 4 Types of Effective Business Tools for Startups
- 4 Ways Technology Can Improve Your Business
- 5 Indispensable Benefits of Regular Heat Pump Maintenance
- 5 Insurance Products to Help Protect Your Family’s Finances
- 5 Main Sources of Prospects for Your Online Business
- 5 Reasons Why Purchasing an Apartment Is a Great Investment
- 5 Reasons Why Waste Removal Is Important For Your Store
- 5 Tech Advancements in Real Estate
- 5 Ways to Stay Fit in Condo Living
- 6 Key Benefits of Insurance in Business
- 6 reasons you should NOT DIY your website
- 6 Top Ways to Increase Your Home’s Resale Value in 2024
- 6 ways to promote seasonal discounts at your retail store
- 7 Advantages of Process Automation
- 7 common myths about Agile
- 7 Expert Reasons Why Your Machinery Shed Should Have a Skillion Roof
- 7 Popular Reasons To Choose A Custom Cake For Your Special Occasion
- 7 Real Estate Shopping Tips for Beginners
- 7 Things to Consider Before Partnering with a 3PL Company
- 7 Ways to Get Top-Quality Roof Repair Services for Your Melbourne Home
- A Guide on Temporary Buildings
- A New Landlord’s Guide to Basic Financial Reports
- A Year in Review – Evaluating Singapore’s Property Investment Opportunities
- Absolute Markets and its Key Features for Trading
- Adapting to the Digital Age: Maximizing Opportunities in Online Business and Technology
- Affordable Iron Sights: Top Picks for High Performance on a Budget
- All about stock exchanges in the Netherlands
- An answer service is essential for small businesses
- Anonymous Online Crypto Payments
- Be Proficient in Managing Costs and Diminish Expenses – Here is How
- Benefits of Having Car Insurance
- Benefits of Influencer Marketing Agency in 2023
- Best Online Shopping Apps
- Best Strategies For Real Estate Businesses To Speed Up Their Digital Marketing Game
- Best Ways to Reduce the Cost of Your Car Loan
- BITCHIP (BTP) Achieves Unprecedented Reliability with Cyberscope’s Rigorous Audit
- Bitcoin Resilience – A Reason to thank HODLers?
- Bitcoin Returns to the Role of Insurance Against Inflation
- BoC Cuts Rates as Inflation Settles into Target Range
- Breaking Down Data Breaches: Causes, Impacts, and Solutions
- Breaking Down the Latest Trends in Affiliate Marketing and Arbitrage
- Buying a Company Car: Key Considerations
- Can You Day Trade Forex Markets?
- Claim You Can Get With the Help of a Car Accident Attorney
- Comparing Rental Properties vs. Flipping Houses in Singapore
- Comprehensive Tips for Sourcing LED Strip Lights from Manufacturers
- Custom ID Badges That Stun: Create Yours Online Today
- Customer Lifetime Value(Clv): What is It and How to Calculate It?
- Cyber-Attack Recovery: Strategies to Get Your Business Back on Track
- Debunking Myths About Outsourcing Sales: The Truth About What It Takes to Succeed with Outsourcing Sales
- Develop a Robust Sustainability Strategy for your Business to Thrive
- Difference between Business Transformation Consultants and strategists
- Discovering the Best Las Vegas Exhibit Companies for Unforgettable Trade Show Experiences
- Ditch the Boardroom: Effective Team-Building Activities to Build Morale
- Does Crypto Have a Negative Impact on the Environment?
- Don’t Derail Your Future Because of an Alleged Academic Misconduct: Hire a Lawyer!
- Dubai Real Estate Market Set for Growth as Renters Eye Homeownership
- Effective Strategies for Growing Your Small Business
- Empowering Girls to Lead SCGS, Orchard Boulevard Condo Offers Top Education and Community Impact
- Essential Steps to Finding the Right Water Damage Restoration Service
- Essential Tips for Businesses on How to Get Finances in Order
- Experience Luxury and Elegance with Charleston Limo Service
- Expert Renovation Tips for HDB Flats in Singapore
- Experts Explain: Open Banking
- Exploring Condo Investment Clubs and Syndicates in Singapore
- Factors Regarding Personal Checks
- Finding the Best Sydney Criminal Lawyers for Your Centrelink Fraud Case
- Four Tips on Cleaning Your Condo
- Global Reach: The Role of Bitcoin ATMs in Expanding Cryptocurrency Adoption
- Got Into a Truck Accident? Here Are The Dos and Don’ts!
- Here Are Ways to Improve Your Work Environment and Increase Productivity When Working from Anywhere
- House Versus Apartment: Which One Is Right For You – Experts Answer
- How a Business Address in Australia Helps Your Company Grow
- How Business Travel Providers Ensure Safety and Security for Travelers?
- How can Travel Management Software Boost Your Travel Business?
- How Do Forex Traders Use ISM Data?
- How Do I Improve My Business?
- How Do Tokenized Stocks Affect the Market?
- How Does a Cryptocurrency Work?
- How Kettle Cookers Improve Efficiency in the Professional Kitchen
- How Net Worth Calculators and Financial Apps Can Help You Achieve Your Financial Goals
- How Smart Money Moves Reflect in Option Chains
- How Soft and Hard Skills are Essential for Managing an Engineering Team
- How Technical Support Can Enhance Your Business Efficiency?
- How To Approach A Real Estate Company To Develop Your Property?
- How To Choose Labeling Solutions
- How to Choose the Right Liquidity Locker for Your Crypto Project: 2025 Updated Guide
- How To Create an E-Commerce Platform
- How To Get Hired With A Resume For Administration
- How to Get the Best Gutter Guard Installation for Your Home
- How to heck ISO 9001 Certificate Validity?
- How to Help Your Child Learn Financial Skills
- How to Improve Your Employee’s Productivity?
- How to Improve Your Restaurant’s Table Turnover Rate
- How to Improve Your Sales Demo and Close Deals
- How to Invest in Mutual Funds in Kuvera
- How to Liquidate a Company in Estonia?
- How To Make Your Furniture Business Stand Out
- How to Optimize Your Company for Hybrid Work
- How To Organize A Cross Country Move
- How to Plan Ahead for a Career Change
- How to Redeem Your Cryptocurrency Bonus: A Comprehensive Guide
- How To Reduce Slips, Trips, And Falls in Your Business
- How to Responsibly Manage Your Company Data
- How to Save Money on Your Favorite Tech Products in Australia
- How to Save Money on Your Fuel Bill
- How to Shop For Clothes on a Budget
- How to Start a Cryptocurrency Exchange
- How to Streamline the Documentation Process for Property Loan
- Hydrovac Excavation: The Evolution of Safe and Efficient Digging Techniques
- India – The Oasis in the Desert for the Stock Market?
- Inflation Report – Possible Surprises for the US Stock Market?
- Injuries With Delayed Symptoms Following A Car Accident
- Investing in Condos with Deferred Payment Schemes (DPS)
- Investing in Condos with Lifestyle Amenities in Singapore
- Is Bitcoin Still a Viable Investment Option?
- Is It Ever Too Late to Get a Lawyer?
- Is Microsoft’s Ban on Crypto Mining in Cloud Services a Reason for Miners to Worry?
- Is Your IT Support Company Letting You Down?
- KuCoin Review – How the KuCoin Bot Can Help You Get the Most Out of Your Crypto Portfolio
- Law Firm Technical Services: What You Need to Know
- Licenses for EMI Structures in Lithuania
- List of Gemstone Names by Color and Type
- Locate Parcel From All Around The World With Ordertracker
- Lucrative Social Media Jobs
- Maximizing Brand Value: Why Every Brand Needs to Monitor MAP
- Memecoins Make a Comeback Despite Crypto Downturn
- Modern Home Design Trends in Singapore for a Chic Look
- Moissanite – the New Diamond?
- Money Management Strategy for Low-Income Earners to Become Rich
- Mortgage Interest Rate Predictions for April 2023
- Myths about Payroll Management Systems That Are Keeping You From Growing
- Norwood Grand Woodlands Gateway to Convenience and Connectivity on Woodlands Avenue 2 and 5
- Online Trading
- Procedures for Payroll in Small Businesses
- Pull Up Banners
- Quantitative Finance: A Deep Dive into Algorithmic Trading
- Questions to Boost Remote Employees’ Productivity
- Reasons Why You Should Trust a Personal Injury Attorney
- Recession Fears – Is the Stock Market Crash Inevitable?
- Role of Influencer Marketing Agencies in UAE for Driving Sales
- Sales Training Programs: The Best Business Investment a Firm Can Make
- Save2be: Pioneering Seamless and Effortless YouTube Video Downloads
- Seaside Splendor: Exploring Italy’s Diverse Beachfront Luxury Real Estate
- Should You Consider a Truck Dispatcher Course?
- Should you include branding with your corporate gifts?
- Singapore Home Makeovers – Expert Ideas to Refresh and Update Your Space
- Singapore Real Estate 2024: Emerging Trends to Watch for Savvy Investors
- Singapore’s Most Popular Home Renovation Design Styles Revealed
- Space-Saving Furniture Ideas for Singapore Apartments
- Spacious and Stylish: Open-Concept Renovation Ideas in Singapore
- Sustainability Steps Businesses can take Today for a Greener Tomorrow
- The A-Z of Home Renovations in Singapore – Comprehensive Tips and Inspiration
- The Advantages of Outside Financial Advice
- The Complete Guide for Commercial Real Estate
- The Complete Guide to Business Telemarketing Services
- The Future of Money: What to Expect from the World of Finance in the Coming Years
- The Future Of NFTs
- The Hidden Risks of Ignoring Leaky Faucets
- The Impact of Economic Indicators on Stock and Share Results
- The Impact of Interest Rates on Singapore Home Loans
- The importance of building a reliable data center infrastructure for business
- The Importance of Security Features in Condo Investments
- The most Effective Technique to Correctly Utilize Low Tack Transfer Tape for Plastic
- The most notable benefits of social media marketing according to an agency in Budapest
- The Pros and Cons of New Developments vs. Resale Properties in Singapore
- The Real Estate Market in Charles County, MD: A Study in Adaptation
- The Rise of Contactless Payment Apps in Small Businesses
- The Rise of Smart Cities: Singapore’s Vision for Urban Innovation
- The Role of Credit Score in Getting a Business Loan Online
- The Sen Condo’s Perfect Location Journey Across Singapore with Ease via the Pan Island Expressway (PIE)
- Things to Consider Before Selecting a B2B eCommerce Software
- Things to Consider When Hiring a Truck Accident Attorney
- Timeless Modern Home Design Trends in Singapore for a Chic and Stylish Look
- Tips for successful trading
- Tips to Maintain Your Credit Balance
- Tips to Transform Your Condo Space into a Tranquil Oasis
- Top 4 Approaches to Quantitative Research in Business
- Top 5 Skills You Will Learn When Becoming a Real Estate Agent
- Top 5 Tips for Starting a Healthcare Business and Making It Grow
- Top 8 Pet-Friendly Communities in Dubai
- Top Benefits of Hiring a Product Liability Attorney for Faulty Products
- Top Benefits of Outsourcing Link Building to Virtual Assistants
- Top Excel Consultants: How to Find the Right Expert for Your Business
- Top Features of an Online Share Trading App to Analyze Idea Share Price Easily
- Top Influencer Marketing Agency for Top-notch Marketing Services
- Top Influencer Marketing Agency in the UAE 2024
- Top UGC Agency in India
- Trading 101: A Beginner’s Guide to Mastering the Basics
- Transformative Impact of the URA’s Vision for Parktown Residences Tampines on Quality of Life and Investment
- Trenchless Pipe Repair: A Cost-effective and Efficient Solution for Plumbing Issues
- Types of Barcodes Scanners& Reasons to Acquire a Holster
- Unlock Agile Marketing with Ad Hoc Reporting
- Unveiling the Power of Investment: Why Your Website Needs Premium Server Hosting
- User Research Tips, Insights, Methods, Benefits & Tools
- Ways You Can Violate A Restraining Order
- Weathering the Waves: The Durability and Versatility of Custom Floating Dock Solutions
- What are Industrial Lifting Bags: features of choice
- What Are Temporary Buildings?
- What are the Advantages of Hiring a Property Manager?
- What are the Best Ways of Empowering Employees?
- What Does the First Half of 2023 Look Like for the Stock Market?
- What Is Customer Identity and Access Management, and How Can It Protect Your Business?
- What Is Fleet Maintenance? 4 Benefits of Fleet Maintenance
- What Is Futures Market Making?
- What Should You Know Before Exploring Original Pukhraj Stone Jewelry
- What to Do When Filing a Los Angeles Personal Injury Lawsuit
- What To Look For When Buying A Refurbished Phone
- What to Look for When Renting a Commercial Warehouse
- When Should a Small Business Consider Outsourcing?
- When Your Home Needs More Than a Roofing Patch
- Why a Secured Loan Could Be Better Than Remortgaging
- Why Custom Web Apps are Good for E-commerce Businesses
- Why do companies need an executive sales recruitment agency?
- Why Do Custom Packaging Boxes Matter For Your Business?
- Why do small businesses need accountants?
- Why is Instagram promotion necessary for your business?
- Why Managing Your Cryptocurrency Portfolio Is The Only Skill You Really Need
- Why Open an Online Savings Account?
- Why We Need An Auto Shipping Expert
- Why you should consider a crypto tracker app
- Why You Should Consult A Lawyer Following A Truck Accident?
- Why You Should Hire an Accident Attorney
- Why You Should Order Items in Bulk
- Will the Ongoing Cardano Improvements Have an Effect on its Price?
- With A Burgeoning Turnpike System Retail Real Estate in Florida Getting a Big Boost
Entertainment
- “The Art of Game Design: Exploring the Creativity Behind Video Games”
- Must-Have Games for the Nintendo Switch 2
- Relaxing Apps While Preparing For a Condo Move
- Explaining Gaming Strategy for Online Gameplay
- 10 Effective Ways To Earn In The Metaverse
- 10 Facts to Research about a Sports Team
- 20 Must-Check Christmas Costumes for Toddlers
- 3 Benefits of Online Game Level Boosting
- 3 Best Things for Fun and No Thoughts
- 3 Cities in Texas You Have to Visit
- 3 Cool Trends To Diversify Your Experience
- 3 Current Trends in Online Games
- 5 Games from NetEnt to play for free
- 5 Golden Rules to be Successful at Online Dating
- 5 Life Lessons Online Games Can Teach You
- 5 Sure Signs Of A Good Crypto Game: Your Simple Guide To Safe BTC Gaming
- 5 Tech Tips to Become a Better Gamer
- 5 Things to Take into Account Before Kitchen Renovation
- 5 Tips To Go On A Holiday When You Have A Pet
- 6 Skills Online Players Must Learn
- 7 Careers That Appeal to Nomadic Lifestyles
- 7 Essential Rules of Online Gaming
- 7 Facts about Online Tournaments
- 7 Popular Red Tiger Games on Autobola Site
- 7 Reasons for Gaming with Bitcoin
- 7 Secrets to a Successful Gaming Session
- 7 Steps to a Successful Tournament
- 7 things you must know before dating a gamer
- 8 Best Cryptocurrency Games and Their Key Features
- 918Kiss Download: 10 of the Most Popular Games You Can Play on the App
- A Comprehensive Guide to Online Gaming In Australia
- A Look Back at the MCU Films of 2021
- A Simple Guide To Fairness In Online Games: RNG And Provably Fair Explained
- All about Airbnb Regulation in France in 2020
- All That You Need To Know About Online Gaming
- All You Need to Know About Online Gaming: Ultimate Guide
- American Video Game Regulation – What Gamers Should Know
- AML Crypto Check Guide
- Amplify Your Podcast’s Reach With Transcription: Unlocking the Benefits of Accurate and Accessible Text Versions
- Are money-based games a good way to have fun?
- Awards, Bonus Codes, And Other Deals on Top Online Gaming Platforms
- Benefits Of Hiring A Video Game Development Agency
- Benefits of Using Litecoin for Online Gaming: What Will You Win?
- Best Entertainment Options With Lockdowns Eased
- Best Free PDF Editor Wondershare HiPDF: Exploring Its AI Features
- Best Gaming Platforms Offering Free Perks
- Best Music Recording Microphones So Far
- Best Rules And Tactics For Games
- Best source of internet entertainment
- Best tour golf occasions Direct
- Beyond Entertainment: Educational Video Games and Their Cognitive Benefits
- Beyond Hospitalisation: Best Medical Insurance with OPD Coverage
- Building a Killer Machine – Key Components of Extreme Gaming PCs
- Building Immersive Worlds: The Role of Environment Design in Online Games
- Can You Make a Career Out of Esports?
- Choose the best one for your relaxing
- Creating the Ultimate Player Experience: Trends in Online Game Design
- Crypto Games: A Complete Guide For Newcomers
- Crypto Gaming Insights: What to Expect in 2022
- Debunking Common Myths About Online Games Websites
- Differences between the various versions of the game
- Different Types of Streaming Video Cameras Available
- Discover the Magic Beyond the Walls: Inside Look at the Spectacular Gaming Castle Sister Sites!
- Discovering the High-Tech World of Virtual Gaming
- Electronic Components Help Gaming Hardware Leap in Performance
- Esports Diversity and Inclusion: Breaking Stereotypes and Building a More Representational Industry
- Essential SEO Tips To Improve Your Online Gaming Site
- Ethical Concerns in Digital Communication
- Evaluating Online Games for Fairness and Security
- Everything Available: Regarding N1 Platform
- Everything You Need to Know About Instagram Addiction
- Everything You Need to Know to Get Ready For the Street Fighter 6 Release
- Exploring the Role of Artificial Intelligence in Online Gaming
- Exploring the Viability and Ethical Considerations of Buying Views for YouTube Technology Review Series
- Fantasy Football Games: Free to Play on Android
- Fashion Forward: The Influence of Online Gaming on Digital and Real-World Fashion
- Feedback Loops in Online Game Design: Player Engagement
- Five excellent benefits of online gaming
- Free Games with Free Coins for More Playtime
- From Casual to Competitive: How to Level Up Your Online Gaming Sessions
- Game Rewards That Keep Giving: From to Massive Games
- Games for Beginners
- Gamification in Online Gaming: Achievements, Levels, and Rewards
- Gaming industry could be worth $320 billion by 2026, says PWC
- Gaming Performance Boosters: A Natural Approach to Focus & Alertness
- Gaming Safely Online: How to Make Sure You Stay Protected
- Gaming Synergy: The Symbiotic Relationship of Esports and Online Games
- Getting the Most Out of Your Spotify Experience
- Gift Ideas That Promote Relaxation
- Harmonizing Wins: Exploring the Connection Between Music and Online Game Success
- Here are Top 8 Tips to Research a Reliable Online Gaming Site
- How Crypto Changed the World of Gaming
- How Enzo Zelocchi is Turning Medicare On Its Head
- How Live Gaming Experiences are Changing the Way People Interact Online
- How Online Gaming Platforms Are Changing The Gaming Landscape
- How Technology Has Changed Online Gaming
- How Technology is Changing Online Games
- How The Computer Games Industry Is Embracing AI
- How the CZUR Starryhub is Revolutionizing Remote Meetings for Hybrid Teams?
- How to Blur Background of Photo on Windows, Mac and Online?
- How to Choose a Gaming Website: A Guide for Novices
- How to Choose the Right Online Game
- How To Excel At Online Video Games
- How to Get Coins for Online Gaming
- How to Host a Bridal Party
- How to Know If Your Site Is Safe for Online Gaming?
- How To Plan The Perfect Silent Disco Party
- How to Play Like a Pro? Check Out 5 Pro Tips
- How to Play Online Games Like a Pro: Tips and Tricks
- How to Play Online Games Safely and Avoid Scams
- How to Prepare Your Condo for Housewarming
- How to Reduce Lag in Modern Warfare 2
- How To Repair MP4 File with Best Video Repair Tool
- How to Stream Entertainment Games
- How to Take Advantage of Online Game Loyalty Programs and VIP Rewards
- How to Use Color to Decorate Your Home?
- How To Win In Online Game in 9 Easy Steps
- How to Win Playing Top Online Games
- How Virtual Reality is Enhancing the Online Game Experience
- Igsnap: Redefining Instagram Interaction for Android Users
- Impact of Advanced Technology on Various Games Using Cryptocurrency
- Improve Your Soccer Gaming with Expert Tips: Soccer Gaming Tips Basics
- Inside the World of Mobile Gaming: Insights on Players and Their Gaming Habits
- Interactive Gaming Challenges: How Missions and Rewards Change the Game
- Interesting Trends to Watch in Gaming
- Introduction to Reels
- Is VR the Future of Gaming?
- Know About The Different Types of Games online
- Local Flavors in Global Arenas: How Cultural Elements Influence Indonesia’s Favorite Online Games
- Make Money with IPL Cricket
- Marketing Mavericks: Rise to Prominence in the World of Online Gaming
- Mastering the Art of Selecting Your Ideal Gaming Destination
- Metaverse and Gaming: How VR is Creating Persistent Virtual Worlds
- Mind Games: The Psychological Benefits of Playing Video Games
- Mobile Gaming – The Future of Online Games
- Must-Have Evening Picnic Essentials
- New Options in Sports Entertainment
- Novice-Friendly Games: How to Find them Online?
- Online gaming: The place where dreams turn into reality
- Outstanding Entertainment And Gaming Platforms In Africa
- Prominent Game Testing Trends that Will Likely Shape the Coming Years
- Psychometrics of Play: Understanding Player Behavior in Online Gaming
- Puzzle Solving and Adventure: The Magic Formula of Treasure Hunt Games
- Reaping Big Rewards with Crypto Gaming
- Residential Proxies improve YouTube experience
- Safe Ways of Cashing out From Online Games in India
- Safety and Security Tips for Online Games Players
- Saudi Arabia Gears Up to Host the 2024 Esports World Cup
- Should You Get an iOS or Android Phone for Gaming?
- Simple Steps to Recover Unplayable and Inaccessible Videos
- Sizzling Seven’s Showdown: Mastering Triple 7 Treasures
- Some most important things you can do to protect yourself and your gains
- Sorare, MLB NFTs: Everything You Must Know
- Staying Ahead of the Game: Mobile Gaming Challenges, Trends, and Opportunities in 2022
- Staying Comfortable for Long Gaming Sessions
- Staying in the Know: A Look at the Top Gaming Trends for 2023
- Tapping Into the Future: Key Trends and Predictions in Mobile Gaming
- Teamwork and Tactics: Strategies for Success in Multiplayer Treasure Hunt Games
- The Art of Spinning: Mastering Game Online for Fun and Profit
- The benefits of playing online games
- The Benefits of Reading Manga in Toonily: A Guide to Help You Fall In Love With Comics Again!
- The Best Way to Level Up in League of Legends: Looking for Gamers
- The Business of Soccer in Mexico: Economics and Sponsorships
- The Engine Behind the Avatar: Exploring Game Development Technologies
- The Esports Explosion: An Analysis of Its Meteoric Rise in the Business World
- The Future of Augmented Reality: How AR Enhances the Treasure Hunt Gaming Experience
- The Future of Flavour: Why Prefilled Pods Are Taking Over in 2025
- The Future of Online Gaming: Exploring Emerging Technologies
- The History of Game Machines: From One-Armed Bandits to Online Games
- The History of Tapestries
- The Impact of Music on Online Game Engagement
- The Impact that Street Fighter II Has Had on the Fighting Game Genre
- The Importance of Using a VPN While Gaming & Watching Your Favorite Sporting Events
- The Increasing Impact of Social Media on News Consumption
- The Latest Technology Trends in Sweden for Norwegian Gaming Sites
- The Power of Entertainment: Creating Relaxation and Connection in a Fast-Paced World
- The Prospects of Play-to-Earn NFT Game Development in the Current Crypto Scene!
- The reliable Joseph-Antoine Bell
- The Role of Artificial Intelligence in Online Gaming Design
- The Secret to Adding More Excitement to Any Sports
- The Technological Evolution of Online Gaming
- The Ultimate Guide to Spotting the Best Online Games
- The Use of Cryptography in Online Games: Ensuring Integrity and Fairness
- Tips for a Hassle-Free Online Games Installation: Your Complete Guide
- Tips for Creating and Editing Entertaining Videos using Movie Maker
- Tips for Identifying Premium Games Online
- TOP 5 CDN Services for Game Apps with Global Audiences in 2023
- Top 5 Classic Games Variations to Try Out
- Top 5 Cyber Sports Trends
- Top 5 Iconic Games to Play
- Top 5 RPG Video Games to Play in 2023
- Top Reasons to Say Yes to Royal TV for Sports Fans
- Top Trends in Crypto Gaming
- Top Video Production Company In India
- Top Video Production Company in India
- Transform Your YouTube Channel: The Ultimate Guide to Using Filmora’s AI Thumbnail Creator!
- Try Your Luck – Winning Strategy
- Understanding How Online Bookmakers Set Odds in 2023
- Understanding the Different Types of Games Available in Austrian Market
- Unlocking the Secrets of Timeless Online Games Popularity
- Unveiling Advanced Marketing Tactics: The Online Game Approach
- Valorant Ban Waves – From Detection to Ejection
- Warzone Cheats Everything you need to know
- Watch and Win: Unlocking the Secrets of Online Gaming Bonuses
- Ways to Stay Safe While Playing your Favourite Video Game
- What are the Online Gaming Business Opportunities Available for Entrepreneurs?
- What Is Aviator Crash Game And How To Play It?
- What to Play Online When I Am Stuck at Home
- When Should You Provide Your Phone Number to Websites?
- Where Should Banjo & Kazooie Go Next?
- Which App is Considered Essential for Every Cricket Enthusiast?
- Why a Massive Following on Facebook is Essential
- Why Are Online Games Gaining Popularity Today?
- Why Are Video Games So Popular and How to Play Them
- Why Do People Love Intense Action Movies?
- Why does Hulu have so many Ads Even though I Pay for my Subscription?
- Why Reviews Are Important: A Gamer’s Perspective
- Why You Should Play Fantasy Cricket?
- Why Your Smartphone is the Ultimate Online Gaming Companion
- Winning Strategies For Online Games
- With online games, you may increase your passive income
- Women’s Rise in the Game of Golf
- ZTE Redefines Home Entertainment with 4K DVB Zapper Set Top Box
News
- Build Your Dream Website in Minutes: The Power of AI Website Builders
- Decoding Posture: Enhancing Spinal Alignment with Posture Bra
- How to Unformat an SD Card on Computer?
- “The Art of Game Design: Exploring the Creativity Behind Video Games”
- [2023 Best Picks] 6 Voice Changers for Discord on Mac
- How to Generate GST Invoices in Seconds with the Right Software
- Which Broadband is Best for Business?
- 5 Tech Tips to Make Your Condo Move Easier
- Best Blockchain to Create a Crypto Token
- Boulevard Coast Jalan Loyang Besar EC A Hub of Convenience and Vibrancy for Residents with a Variety of Retail and Recreational Options
- How to Choose the Best Law Firm
- How to Create a Quick Moving Checklist
- Must-Have Games for the Nintendo Switch 2
- Relaxing Apps While Preparing For a Condo Move
- Singapore’s Real Estate Investment Strategies for Savvy Families
- The 4 Different PC Cases and a Brief Guide on What to Buy
- Tips to Get More Natural Light in Your Home
- What Are the Eligibility Criteria for Low-Interest Personal Loans?
- Why Comprehensive Bike Insurance is Becoming a Must-Have in Urban India?
- 4 Reasons Why You Should Hire Digital Nomads
- Best Paid Survey Site to Make Extra Money
- Explaining Gaming Strategy for Online Gameplay
- Exploring the New Frontier of Design and Technology with Sarah Zaheer
- How Consumers Can Leverage Big Data
- How to Scale Your Software Development Team with Team Augmentation?
- Novelfull: A Must-Visit Free Reading Platform
- SEO Analytics: Tracking Your Results
- Trenchless Pipe Repair: An Efficient and Economical Plumping Solution
- 10 Awesome Video Types to Promote Your Business
- 10 Best Mobile Application Testing Tools in 2023
- 10 Best Practices for eCommerce Website Security
- 10 Books To Scale Up And Build A Better Company
- 10 Effective Ways To Earn In The Metaverse
- 10 Facts to Research about a Sports Team
- 10 Reason You Should Never Attempt To Detox At Home For An Addiction Problem
- 10 soft skills that recruiters are looking out for today
- 10 Unique Dropshipping Products to Sell in 2022
- 11 Packaging & Label Design ideas Help your Business Growth
- 14 Tips and Tricks to Optimise Your Laptop for Gaming
- 20 Must-Check Christmas Costumes for Toddlers
- 20 Ways to Have a Brilliant Stag Weekend in Cardiff
- 2023 Forecast – What’s in Store for EUR/USD?
- 3 Benefits of Online Game Level Boosting
- 3 Best Things for Fun and No Thoughts
- 3 Cities in Texas You Have to Visit
- 3 Cool Trends To Diversify Your Experience
- 3 Current Trends in Online Games
- 3 Keys to a Successful Corporate Presentation
- 3 Major Credit Card Scams & How to Avoid it
- 3 Tech Startup Ideas to Look into
- 3 Ways HVAC Software Can Improve Your Productivity
- 3 Ways to Enter the IT Market
- 3 Ways to Make Hiring Easier in a Still-Tight Labor Market
- 4 Advantages of Buying Bitcoin Now
- 4 Benefits of Using a Professional Plumber for Your Bathroom Remodel
- 4 Best Ever Applications to Hide your Photos & Videos
- 4 Devastating Ways Blocked Gutters Can Damage Your Home
- 4 Essential Things You Can Do Online After Moving House
- 4 Ideas to Spice Up Your Marketing Strategy
- 4 Key Benefits of Hosting Quickbooks On the Cloud
- 4 Methods: How to Unlock Android Phone Without Password
- 4 Obvious Benefits of Professional Carpet Cleaning
- 4 Reasons Why You Need High-Performance Inserts in Metalworking
- 4 Tech Tips for Organizing Your Condo Space
- 4 Tech Tips to Find the Best Condominium Online
- 4 Technologies Used in Green Construction
- 4 Tips for Making Your Online Marketplace Run Smoother
- 4 Tips for Managing the Online Reputation of Your Small Business
- 4 Tips for Navigating Menopause
- 4 Tips to Build Your Demand Gen Marketing Strategy for 2022
- 4 Tips to Choose the Best Location For Your Next Home
- 4 Tips to Help You Transition from ADSL Broadband; It Could Be Coming to an End
- 4 Tips to Land a Role in the DevOps Industry
- 4 Tips to Maximize Your Living Area Space
- 4 Tips When Shopping for a Potential New Home
- 4 Top Sustainable Practices in Geotechnical Engineering Worth Implementing
- 4 Types of Effective Business Tools for Startups
- 4 Warning Signs that You Should Urgently Call an Emergency Electrician
- 4 Ways QR codes are used in Marketing
- 4 Ways Technology Can Improve Your Business
- 4 Ways to Increase Your Vitamin Intake
- 4 Ways to Prep Before Going to The Polls
- 4 Ways to Protect Borrowers’ Personal Information
- 5 Advantages of the Internet for Businesses and Students
- 5 Amazing Benefits of Native Plants that Your Landscaper Wants You to Know
- 5 Applications of Peptides in Industrial Biotechnology
- 5 apps that can compromise your privacy big time
- 5 Benefits of a Condo in a Work From Home Setup
- 5 benefits of Cloud Software for Medical Practices
- 5 Benefits Of Data Analytics With Modern Tools
- 5 Best Low-Investment Business Ideas You Can Start Online
- 5 Best Practices for Affiliate Email Marketing Campaigns
- 5 Clear Warning Signs that You May Need a Root Canal
- 5 Crucial Applications of Pedigree Manager Software in Pigeon Racing
- 5 Easy Tech Tips to Secure Your Home
- 5 Essential Accessories for Custom Profile Extrusion
- 5 Exclusive Tips To Create Google Friendly Content
- 5 Expert Reasons Why Specialised Soil Testing is Essential Before Any New Build
- 5 Facts Why working from home doesn’t work for everyone
- 5 Fun Indoor Toys for Your Pet Cat
- 5 Games from NetEnt to play for free
- 5 Golden Rules to be Successful at Online Dating
- 5 Guaranteed Ways To Streamline Remote Hiring for Recruiters
- 5 Home And Natural Remedies For Your Toothache Pain
- 5 Indispensable Benefits of Regular Heat Pump Maintenance
- 5 Insurance Products to Help Protect Your Family’s Finances
- 5 Key Factors That Determine Your Housing Loan Eligibility
- 5 Life Lessons Online Games Can Teach You
- 5 Lucrative Online Jobs That Can Skyrocket Your Income
- 5 Main Sources of Prospects for Your Online Business
- 5 Mistakes to Avoid in your Presentation
- 5 Oklahoma-Specific Red Flags in Car Accident Settlement Offers
- 5 Organization Tips for the Soon-to-Be College Freshman
- 5 Products That Can Make Your Home Smell Better Fast
- 5 Realistic Ways to Make Money Online in 2023
- 5 Reasons to Shop for Properties Online
- 5 Reasons Why More People Today Choose to Read
- 5 Reasons Why Purchasing an Apartment Is a Great Investment
- 5 Reasons Why Waste Removal Is Important For Your Store
- 5 Reasons Why You Should Use a VPN for Online Security
- 5 Rewarding Career Paths 2023
- 5 Sure Signs Of A Good Crypto Game: Your Simple Guide To Safe BTC Gaming
- 5 Surefire Ways to Prepare for a Social Media Interview
- 5 Tech Advancements in Real Estate
- 5 Tech Devices for Cleaning Your House Efficiently
- 5 Tech Tips to Become a Better Gamer
- 5 Things to Take into Account Before Kitchen Renovation
- 5 Tips on Setting Up Your Condo’s Wi-Fi Network
- 5 Tips to Create a Smooth-flowing Chart
- 5 Tips To Go On A Holiday When You Have A Pet
- 5 Top Benefits of Upgrading Your Home’s Electrical Switchboard
- 5 Top Tips to Keep in Mind When Choosing New Tapware
- 5 Top Ways an Electric Lift Chair Can Help with Mobility Issues
- 5 Useful Things Local SEO Experts Can Do for Your Company
- 5 Ways Having a Smaller Space Can Benefit You
- 5 Ways Magento PWA Studio Will Transform eCommerce in 2024
- 5 Ways Tech-Minded People Can Be Successful in Their Industry
- 5 Ways To Find The Right Buyer For Your Dental Practice
- 5 Ways To Increase Your Confidence
- 5 Ways to Prepare for a Sick Day at Home
- 5 Ways to Stay Fit in Condo Living
- 5 Ways to Use Technology for Moving House
- 6 Content Marketing Ideas for Small Businesses
- 6 Expert Reasons to Invest in a Pest Inspection Before Buying Your Dream Home
- 6 Key Benefits of Insurance in Business
- 6 Must-Have Home Appliances in 2024
- 6 Must-Have Items for the New Condo Dweller
- 6 NFTs that Run on the Solana Blockchain
- 6 Personal Tech to Stay Cool and Comfortable
- 6 Plants You Need to Grow for Sleep, Anxiety, and Calmness
- 6 Possible Signs You May be Heading for Dentures
- 6 Professional Tips to Help You Budget for Kitchen Equipment Maintenance
- 6 Reasons Why Sports Organizations Need Digital Media Distribution
- 6 Reasons Why the Surviving Family Must Take Legal Action Against the Negligent Party
- 6 reasons you should NOT DIY your website
- 6 Skills Online Players Must Learn
- 6 Steps to Launch a Profitable Online Course
- 6 Steps to Successfully File Returns Without Form 16
- 6 Things to look for when selecting the right HTML5 Web-to-Print Software
- 6 Tips to Follow for Vue js Deployment Practices
- 6 Top Ways to Increase Your Home’s Resale Value in 2024
- 6 ways to promote seasonal discounts at your retail store
- 7 Advantages of Process Automation
- 7 Best SEO Tools According to Expert SEO Strategists
- 7 Careers That Appeal to Nomadic Lifestyles
- 7 common myths about Agile
- 7 Easy Ways to Pamper Yourself During Weekend
- 7 Essential Rules of Online Gaming
- 7 Expert Reasons Why Estate Planning Should Be On Your To-Do List in 2024
- 7 Expert Reasons Why Your Machinery Shed Should Have a Skillion Roof
- 7 Facts about Online Tournaments
- 7 Health Products That Help to Improve Your Overall Well-Being
- 7 Key Components to Include in a Risk Management Plan
- 7 Must-Have Gadget Accessories
- 7 Popular Reasons To Choose A Custom Cake For Your Special Occasion
- 7 Popular Red Tiger Games on Autobola Site
- 7 Proven Strategies for Growing Your Veterinary Practice (2024)
- 7 Real Estate Shopping Tips for Beginners
- 7 Reasons for Gaming with Bitcoin
- 7 Reasons to Choose a Smart Thermostat
- 7 Reasons Why Poor Mental Health Is The Leading Cause Of Addiction
- 7 Secrets to a Successful Gaming Session
- 7 Steps to a Successful Tournament
- 7 Things to Consider Before Partnering with a 3PL Company
- 7 things you must know before dating a gamer
- 7 Tips for a Seamless Move to a Condo
- 7 Ways to Get Top-Quality Roof Repair Services for Your Melbourne Home
- 7 Ways to Speed Up Your Old iPhone In Under 5 Minutes
- 8 At-Home Anxiety Reduction Techniques To Master Peace
- 8 Benefits of Automating Manual Hospital Processes
- 8 Best Cryptocurrency Games and Their Key Features
- 8 Best Essential Security Features That Every Ecommerce Website Should Have
- 8 Easy Steps To Get To Know Your Natural Weight Loss System
- 8 Emergency Plumbing Situations that Call for an Expert Plumber
- 8 Must-Visit Places in Rajasthan & Best Time to Visit Them
- 8 Personality tests You Should Take Before Applying For A Job
- 9 Steps To Developing a Language Learning App
- 918Kiss Download: 10 of the Most Popular Games You Can Play on the App
- A 1-Click Phone Data Transfer Method for Android to iPhone
- A Comprehensive Guide to Creating a Strong HealthTech Marketing Strategy
- A Comprehensive Guide to Online Gaming In Australia
- A Comprehensive Guide To Understanding Credit Cards: Read This Before Applying!
- A Guide on Temporary Buildings
- A Guide To 3D Automated Optical Inspection
- A Guide to Shopping for Health Products When You’re a Newbie
- A Guide to Starting a Payment Processing Company
- A Look Back at the MCU Films of 2021
- A New Landlord’s Guide to Basic Financial Reports
- A Simple Guide To Fairness In Online Games: RNG And Provably Fair Explained
- A Tech Career: Is a B.Tech CSE the first step?
- A Year in Review – Evaluating Singapore’s Property Investment Opportunities
- About Neina
- Absolute Markets and its Key Features for Trading
- Achieving a Cohesive Groomsmen Look
- Adapting to the Digital Age: Maximizing Opportunities in Online Business and Technology
- Addressing Common HVAC Problems in Renton Homes: A Guide to Optimal Comfort
- Advanced Reporting Techniques for Selenium Test Results
- Advancing Efficiency with Power Monitoring and Quality Solutions
- Affordable Iron Sights: Top Picks for High Performance on a Budget
- AI Detectors: Safeguarding Authenticity in the Digital World
- All about Airbnb Regulation in France in 2020
- All About Human Hair Extensions
- All About Snapchat Premium
- All about stock exchanges in the Netherlands
- All That You Need To Know About Online Gaming
- All You Need To Know About Air Tack Mats
- All You Need to Know About Online Gaming: Ultimate Guide
- Amazon is Planning to Launch a Smart Fridge
- American Video Game Regulation – What Gamers Should Know
- AML Crypto Check Guide
- Amplify Your Podcast’s Reach With Transcription: Unlocking the Benefits of Accurate and Accessible Text Versions
- An answer service is essential for small businesses
- An Overview of C++: Learn About Polymorphism, Constructors & more
- Anonymous Online Crypto Payments
- API Security Becomes a Growing Concern Amid Explosive Growth
- API Testing and Why It’s Essential
- Apple Number 1 in Smartphone Sales for Q4 2021
- Are frequent meetings with employees necessary? Work efficiency and what affects it
- Are iPhone 15s Pushing Mobile Gaming Developers to New Levels?
- Are money-based games a good way to have fun?
- Are Peptides the Same as Proteins?
- Autoclave for hospital equipment – What should I know before buying one?
- Avica Remote Desktop Review: Secure, Simple and Seamless
- Avoiding Common Pitfalls When Renovating Singapore Homes: Essential Ideas
- Awards, Bonus Codes, And Other Deals on Top Online Gaming Platforms
- Banjo-Kazooie to Arrive on Nintendo Switch Online
- Basis of EMI Licensing in UK
- Battling Substance Abuse And Addiction In Las Vegas: 10 Things You Should Do
- Be Proficient in Managing Costs and Diminish Expenses – Here is How
- Behind the Scenes: Understanding the Role of Parks and Rec Offices in the Community
- Benefits of Automating IT Asset Discovery
- Benefits of Choosing a 3% Cash Back Card
- Benefits of Having a Pet in a Condo
- Benefits of Having Car Insurance
- Benefits of Hiring a Personal Injury Attorney if You Are Injured
- Benefits Of Hiring A Video Game Development Agency
- Benefits of Hiring an Outsource Firm
- Benefits of Influencer Marketing Agency in 2023
- Benefits of Using Litecoin for Online Gaming: What Will You Win?
- Benefits Of Using Token Authentication For Your Security
- Benefits Of Velocity-Based Training For Athletes
- Best cases for Google Pixel 7
- Best Christmas Deals: Tips for a Season of Savings & Giving 2022
- Best Dive Watches for Professionals and Enthusiasts in 2025
- Best Entertainment Options With Lockdowns Eased
- Best file managers for Android: 10 proven options
- Best Free PDF Editor Wondershare HiPDF: Exploring Its AI Features
- Best Free PDF Editor for College Students to Level Up Productivity
- Best Gaming Platforms Offering Free Perks
- Best Hard Drive Recovery Software: Wondershare Recoverit
- Best Health Care Plans- What to Look for and the Various Types
- Best Methods to Protect Your Router from Viruses
- Best Music Recording Microphones So Far
- Best Online Shopping Apps
- Best Practices for Python Developers
- Best Relaxed Hair Extensions for African American Women: What to Look For
- Best Restaurants for Students to Hangout in Melbourne
- Best Rules And Tactics For Games
- Best source of internet entertainment
- Best Strategies For Real Estate Businesses To Speed Up Their Digital Marketing Game
- Best tour golf occasions Direct
- Best way to Transfer Data from iPhone to Samsung Galaxy Series
- Best Ways to Reduce the Cost of Your Car Loan
- Beyond Entertainment: Educational Video Games and Their Cognitive Benefits
- Beyond Hospitalisation: Best Medical Insurance with OPD Coverage
- Beyond the Aesthetics: Rhinoplasty’s Side Effects
- Beyond the Horizon: How Foreman’s Visionary Approach Shapes the Future of Crypto Mining
- Big Brother Era: Is Biometric Espionage a New Reality?
- BITCHIP (BTP) Achieves Unprecedented Reliability with Cyberscope’s Rigorous Audit
- Bitcoin Resilience – A Reason to thank HODLers?
- Bitcoin Returns to the Role of Insurance Against Inflation
- BoC Cuts Rates as Inflation Settles into Target Range
- Boost Office Productivity With These Easy Actions
- Boost Your Home’s Value with These Quick Fixes in Singapore
- Boosting E-Commerce Sales through Accurate Email Address Lists
- Branding 101: Why Your Custom-Printed Marquees Work as Hard as You
- Breaking Down Data Breaches: Causes, Impacts, and Solutions
- Breaking Down the Latest Trends in Affiliate Marketing and Arbitrage
- Bridging the Knowledge Gap: How Retrieval-Augmented Generation Transforms AI Responses
- Building a Killer Machine – Key Components of Extreme Gaming PCs
- Building Immersive Worlds: The Role of Environment Design in Online Games
- Business Applications and Benefits of Professional Outbound Calling
- Buy Real YouTube Subscribers: Buy Organic YouTube Subscribers USA
- Buying a Company Car: Key Considerations
- Bypass FRP Lock on Xiaomi: A Comprehensive Tutorial
- C-Suite Insights: 5 Benefits of Software Outsourcing
- Cabinet Styles That Define Contemporary Elegance
- Camp Lejeune Water Contamination And Its Dangers
- Can I switch from Actual Expenses to Standard Mileage Rate?
- Can Short-Term Lenders Aid in Improving Your Credit Score
- Can Taking THC O Gummies Strengthen Your Immunity?
- Can You Day Trade Forex Markets?
- Can You Make a Career Out of Esports?
- carpet cleaning in a Austin, Texas hospital by The Steam Team of Austin and laundering surgical scrub caps in commercial washing machines
- Carry Your Message: The Versatility of Branded Cotton Tote Bags
- Cashout Fridays Really Legit?
- Challenges For Companies Going Global: What Experts Says
- Choose the best one for your relaxing
- Choosing A Clinic To Treat Mental Health Issues: 7 Things To Know
- Choosing the Right Moving Company for Your Cross-Country Relocation
- Claim You Can Get With the Help of a Car Accident Attorney
- Collection of Watermark Removal Tools: How to Get Rid of Watermarks Across Different Media
- College Student Loans: A Comprehensive Guide
- Combating Climate Change With New Technologies
- Combining sports and cardio for a dynamic workout routine
- Commercial Benefits of Using Solar Rooftop Panels for Businesses
- Common Mistakes To Avoid In Market Research
- Common Mistakes You Should Avoid While Driving a Car
- Company problem solvers
- Comparing Rental Properties vs. Flipping Houses in Singapore
- Comparison between Germany and Singapore (Asia) VPS
- Comprehensive Tips for Sourcing LED Strip Lights from Manufacturers
- Condo Tech Revolution: How Apps Are Changing the Way We Live and Interact
- Conjuctivochalasis: Wrinkled Eyeballs
- Content Euphoria – A Content Writing Company In Bangalore With A Global Presence
- Contraceptive Options: Finding the Right Fit for You
- Could Artificial Intelligence Deliver Cheaper Car Insurance?
- CPUs and GPUs to Get Price Hikes in 2022
- Creating the Ultimate Player Experience: Trends in Online Game Design
- Creating Websites is Not Just About Coding Anymore
- Creating your Own Office Pods
- Cruising Through the Ocean to Rule the Land: Shazmeer Jiwan and His Journey
- Crypto Games: A Complete Guide For Newcomers
- Crypto Gaming Insights: What to Expect in 2022
- Crypto.com Loses $15M in Ethereum in Hack
- Custom ID Badges That Stun: Create Yours Online Today
- Customer Lifetime Value(Clv): What is It and How to Calculate It?
- Cute and practical office helper: Akko Doraemon Macaron 3098B
- Cyber-Attack Recovery: Strategies to Get Your Business Back on Track
- Cyberattack causes major disruption to UK medical helpline ‘120’
- Danger of Hookah Pens
- Dangers of Raw Sewage Under House
- Data Entry Virtual Assistants: Boosting Productivity
- Debunking Common Myths About Online Games Websites
- Debunking Myths About Outsourcing Sales: The Truth About What It Takes to Succeed with Outsourcing Sales
- Decluttering Tips: Embracing the Minimalist Condo Lifestyle
- Decoding Everything About Python vs .NET in 2023 for Businesses
- Defining Appointment Setting Services And the Reasons Behind Outsourcing It
- Demystifying VPN: Exploring the Differences between Personal and Business VPNs
- Dental Implants vs. Traditional Dentures: Which One is Right for You?
- Designing Effective Visualizations for SQL Reports
- Detection of Phenibut in a Commercially General Product in Richmond, VA: A Topic Research
- Develop a Robust Sustainability Strategy for your Business to Thrive
- Difference between Business Transformation Consultants and strategists
- Differences between the various versions of the game
- Different Types of Microphones
- Different Types Of ONYC Hair Clip Ins To Try
- Different Types of Streaming Video Cameras Available
- Digital Life bank Is Building Products, Investing in Climate-Focused Artificial Intelligence Platform
- Digital Marketing- Do’s and Don’ts
- Digitizing High-Volume Document Workflows: Why File-Heavy Teams Need Better eSignature Infrastructure
- Direct Impact or Indirect Gains: The Bitcoin ETF Dilemma
- Discover the Benefits of Mobile apps for Entertainment
- Discover the Brilliance of the OPPO A79 5G Sunlight Display: A Visual Revolution for Mexican Smartphone Users
- Discover The Different Applications Of A Mini Excavator
- Discover the Excellence of Raffles Institution A Short Drive from Toa Payoh with The Orie Showflat
- Discover the Magic Beyond the Walls: Inside Look at the Spectacular Gaming Castle Sister Sites!
- Discovering the Best Las Vegas Exhibit Companies for Unforgettable Trade Show Experiences
- Discovering the High-Tech World of Virtual Gaming
- Disposal of Used Batteries
- Ditch the Boardroom: Effective Team-Building Activities to Build Morale
- DLP and Cybersecurity: Building a Comprehensive Defense Strategy
- Do I Need a VPN in the UAE?
- Do You Need an Instagram Follower Tracker?
- Does Crypto Have a Negative Impact on the Environment?
- Does SUSHI Have High Annual Returns?
- Don’t Derail Your Future Because of an Alleged Academic Misconduct: Hire a Lawyer!
- Download Windows Backup Software: Protect Your Data
- Dr. Sanyasi Naidu Dadi is Bringing Modern Solutions to Underground Resource Management and Natural Disaster Mitigation Platforms
- Driving Under the Influence: Consequences for the Driver
- Dubai Real Estate Market Set for Growth as Renters Eye Homeownership
- Effective payment methods for Google Ads: tips for choosing payment cards
- Effective Strategies for Growing Your Small Business
- Efficient Heating and Cooling Solutions with Single Zone Mini Splits
- El Green Mall: Democratizing the Sustainable Market
- Electronic Components Help Gaming Hardware Leap in Performance
- Elevate Your Server Management: Windows Servers + RDP
- Embarking on the Tech Journey: How Cloud-Based Solutions Propel Small Businesses
- Emotional Design: Creating Products That Forge Deeper User Connections
- Empowering Girls to Lead SCGS, Orchard Boulevard Condo Offers Top Education and Community Impact
- Empowering Success ITE College East – Training Future Professionals at Aurelle of Tampines EC
- Eneba Brings Down God of War PC’s Price to Just $37.76
- Enhancing Connectivity: Innovative Solutions for Today’s IT Challenges
- Ensuring Safety and Peace of Mind with Armed Security Services
- Esports Diversity and Inclusion: Breaking Stereotypes and Building a More Representational Industry
- Essential Insights into Children’s Healthcare Orthopedics: The Ultimate Guide
- Essential Insights into Milk Processing Equipment for Modern Dairy Operations
- Essential SEO Tips To Improve Your Online Gaming Site
- Essential Steps to Finding the Right Water Damage Restoration Service
- Essential Tech Items For Your New Condo
- Essential Tips for Businesses on How to Get Finances in Order
- Ethical Concerns in Digital Communication
- Evaluating Online Games for Fairness and Security
- Everything Available: Regarding N1 Platform
- Everything You Need to Know About Instagram Addiction
- Everything You Need to Know to Get Ready For the Street Fighter 6 Release
- Examples of Good and Bad Spending Habits
- Experience Luxury and Elegance with Charleston Limo Service
- Experience the Best of Freshness at Jurong East Wet Market in Otto Place Parce B
- Experience Ultimate Convenience and Entertainment at Chencharu Close Condo, Adjacent to Northpoint City – Singapore’s Premier Suburban Mall
- Expert Insights on Commercial Concrete Cutting in Sarasota
- Expert Renovation Tips for HDB Flats in Singapore
- Experts Explain: Open Banking
- Exploding Flavors: The Thrill of Chicken Firecracker Cireng
- Exploring Artistic Metalwork in Household Items
- Exploring Condo Investment Clubs and Syndicates in Singapore
- Exploring Data Science with Python
- Exploring New Advancements in Alzheimer’s Disease Treatment
- Exploring The Beauty And Versatility Of Wood Table Tops
- Exploring the Latest Lincoln Subdivisions in Christchurch: A Comprehensive Guide
- Exploring the Role of Artificial Intelligence in Online Gaming
- Exploring the Viability and Ethical Considerations of Buying Views for YouTube Technology Review Series
- Facelift Surgery Trends You Need to Know
- Factors Regarding Personal Checks
- Factors That Affect Car Loan Interest Rates: A Deep Dive
- Factors to Consider When Planning for Your Custom Home
- Fantasy Football Games: Free to Play on Android
- Fashion Forward: The Influence of Online Gaming on Digital and Real-World Fashion
- Feedback Loops in Online Game Design: Player Engagement
- Field Service Management Software: Your Key to Streamlining Business Operations
- Financial Services You Can Find Online
- Finding the Best Sydney Criminal Lawyers for Your Centrelink Fraud Case
- Finding the Right Endometriosis Specialist in Portland: Your Comprehensive Guide
- Five excellent benefits of online gaming
- Five Powerful Project Prioritization Methods
- Five Top Arguments for Hiring Professional Security Guards for Your Business
- Flipbooks: A New Era in Digital Publishing
- Fluoride 101: Facts, Myths, and Common Misconceptions
- Flutter Vs React Native For App Development: Which Is Better in 2022?
- Focus on Amnesia
- Fortifying Your Digital Castle: The Power of ProGuard Security
- Fortifying Your Ride: 4 Essential Tracking Functionalities Every Car Owner Must Utilize to Protect Their Vehicle
- Four Tips on Cleaning Your Condo
- Free Games with Free Coins for More Playtime
- From Brain to Heart: How Dubai Hospitals Offer Comprehensive Care
- From Casual to Competitive: How to Level Up Your Online Gaming Sessions
- From Discovery to Protection: How DSPM Secures Your Data Lifecycle
- From Futon to Fortune: My Journey to Success
- From Obscurity to Stardom: The Rise of Stefan Soloviev
- From Traditional Banking to Online: Savings Account Transformation
- From Virtual to Reality: How Home Design Apps Are Changing the Construction Industry
- Future Trends in Dentistry and How an Online Dental Nurse Course Can Prepare You
- Future Trends of Data Consulting
- Game Rewards That Keep Giving: From to Massive Games
- Games for Beginners
- Games to Play If You Just Want to Chill and Relax
- Gamification in Online Gaming: Achievements, Levels, and Rewards
- Gaming industry could be worth $320 billion by 2026, says PWC
- Gaming Performance Boosters: A Natural Approach to Focus & Alertness
- Gaming Safely Online: How to Make Sure You Stay Protected
- Gaming Synergy: The Symbiotic Relationship of Esports and Online Games
- Garmin Launches Fenix 7 Smartwatch Lineup
- Generate Dynamic QR Code To Have A Flexible Campaign
- Get Better at Blog Writing: 5 Essential Steps
- Getting ISO 9001, ISO 14001, and ISO 45001 as a bundle
- Getting the Most Out of Your Spotify Experience
- Gift Ideas That Promote Relaxation
- Give life to old memories with AI-powered photo restoration tools
- Global Reach: The Role of Bitcoin ATMs in Expanding Cryptocurrency Adoption
- Golf Holidays In Murcia
- Golf Holidays Morocco
- Got Into a Truck Accident? Here Are The Dos and Don’ts!
- Great Ways to Stay Fit and Healthy
- Guide to Creating Digital Marketing Strategy for Small Business
- Guide to Hong Kong’s Employment Visa Requirements
- Guide to visiting Palm Springs
- Hadoop Backup Solutions and Large Data Pools
- Harmful SEO Links – How to Identify Them
- Harmonizing Wins: Exploring the Connection Between Music and Online Game Success
- Headliners Media: Accelerating Growth Online for Startups, SMBs, and Growing Enterprises with PR, Content Marketing & SEO
- Healing from Narcissistic Abuse: Reclaiming Your Inner Strength and Raising Your Self-Worth
- Health Benefits Of Being An Outdoor Person
- Health Benefits Of Using Custom Air Filters
- Health Innovation as Part of a Benefits Strategy
- Healthier Living Through Filtration: Identifying the Premier Choice
- Healthy Snacks You and Your Kids Can Make at Home
- Helpful Tips for Undertaking Your First House Flip
- Here are Top 8 Tips to Research a Reliable Online Gaming Site
- Here Are Ways to Improve Your Work Environment and Increase Productivity When Working from Anywhere
- Here’s why ignoring the Ugly Truth behind Rex Real Estate Is Not a Smart Decision
- Here’s Why Kids Need to Learn How to Code
- Hollandse School Nurturing Dutch Education at The Sen Condo SL Capital
- House Versus Apartment: Which One Is Right For You – Experts Answer
- How a Business Address in Australia Helps Your Company Grow
- How a Home Care Marketing Firm Can Help You Generate More Leads and Grow Your Business
- How AI Apps Are Ushering in a New Era for Product Photography
- How AI Can Help Financial Advisors Grow
- How AI is Transforming Retail with Voice Technology
- How AI Will Help Data Analytics
- How Business Analysis Tools Streamlines Project Success?
- How Business Travel Providers Ensure Safety and Security for Travelers?
- How Cakesicle Decorating Kits Can Help You Create Beautiful Desserts
- How can an Insurance Agent Help in Choosing Affordable Insurance Policies?
- How can Hospitals be a Safe Place, When they’re so Contaminated to be at?
- How can ISO 9001 improve your business?
- How Can Learning A New Qualification Improve My Life?
- How can Travel Management Software Boost Your Travel Business?
- How Climate Affects Your Air Conditioning System in Port St. Lucie
- How Crypto Changed the World of Gaming
- How Data Fabric Can Improve Efficiency and Performance in the Workplace
- How DBT & CBT Therapy Helps with PTSD Treatment
- How Do Forex Traders Use ISM Data?
- How Do I Find Cable Internet in My Area?
- How Do I Improve My Business?
- How Do Payment Methods Protect the Data of Polish Players?
- How Do Tokenized Stocks Affect the Market?
- How do you choose a DWI attorney?
- How Does a Cryptocurrency Work?
- How Does SWP Calculator Help You Adjust Withdrawals Based on Market Conditions?
- How Does the Anti-THC Spray Guarantee your Peace of Mind?
- How Does Website Designing Influence Search Engine Optimization?
- How Dynamic Case Assignment is Elevating Customer Service in the Travel Industry
- How Enzo Zelocchi is Turning Medicare On Its Head
- How Hard It Really Is to Buy and Maintain a Scooter? Is it Worth it?
- How Hunting Can Improve Mental Health and Foster Personal Development
- How IoT technology can make our house smart?
- How is JavaScript Constructed: A Deep Dive into its Origins and Evolution
- How Kettle Cookers Improve Efficiency in the Professional Kitchen
- How Keyless Entry Can Boost Your Home Security Efforts
- How Live Gaming Experiences are Changing the Way People Interact Online
- How Net Worth Calculators and Financial Apps Can Help You Achieve Your Financial Goals
- How Net Worth Calculators and Financial Apps Can Help You Achieve Your Financial Goals
- How Noble’s Innovations in Medication Delivery Are Revolutionizing Patient Care
- How Often Should I Renew My CPR Certification?
- How Often Should You Shampoo Your Hairpiece?
- How Online Gaming Platforms Are Changing The Gaming Landscape
- How Rehab Centers are Important for an Addiction Free Society
- How Remote Developers are Reshaping the Software Industry?
- How Residential Proxies Aid Web Scrapers
- How Smart Money Moves Reflect in Option Chains
- How Soft and Hard Skills are Essential for Managing an Engineering Team
- How Technical Support Can Enhance Your Business Efficiency?
- How Technology Can Help You Stay Focused at Work
- How Technology Has Changed Online Gaming
- How Technology is Changing Online Games
- How The Computer Games Industry Is Embracing AI
- How the CZUR Starryhub is Revolutionizing Remote Meetings for Hybrid Teams?
- How To Ace your CAMS Certificate
- How to Actually Enjoy and Have Fun When Moving to a New House
- How To Approach A Real Estate Company To Develop Your Property?
- How To Avoid Unproductive Meetings At Work
- How To Become A Top Real Estate Agent In 11 Easy Steps
- How to Blur Background of Photo on Windows, Mac and Online?
- How to Browse Safely and Anonymously Online
- How to Choose a Gaming Website: A Guide for Novices
- How To Choose Labeling Solutions
- How to Choose the Best Google Ads Service Provider for Your Brand
- How to Choose the Right Liquidity Locker for Your Crypto Project: 2025 Updated Guide
- How to Choose the Right Online Game
- How to Choose the Right Welding Machine
- How to Choose the Right Yoga Mat?
- How to Collect and Track Payments like a Pro with Virtual Account API
- How to Complete Registration and Receive SMS without a SIM card
- How to Convert Your Digital COVID-19 Vaccination Card into a QR Code?
- How to Create a Successful SaaS SEO Strategy in 2022
- How to create a website design concept in 5 steps
- How to Create Amazing Graphics with Your Smartphone
- How To Create An Avatar With Picrew
- How To Create an E-Commerce Platform
- How to Create Training Videos with AI: A Step-by-Step Guide
- How to Develop Mobile Applications
- How to Draw a Circuit Diagram Easier With EdrawMax
- How To Elevate Mouth Corners
- How to Encrypt Email?
- How to Enhance Safety When Sending Encrypted Online Message
- How To Ensure A Successful Commercial Construction Project
- How To Excel At Online Video Games
- How to Find Best SEO Company in Hong Kong
- How to Find Motivation in Writing Academic Papers
- How to Find Out College Loan Interest Rates?
- How to Find ROI From the Gross Rent Multiplier and Vice Versa
- How to Find the Best Deals on AppHut?
- How To Get A Disposable Virtual Number For SMS Verification
- How to Get a Virtual Office Address for My Company?
- How to Get Coins for Online Gaming
- How To Get Hired With A Resume For Administration
- How to Get the Best Gutter Guard Installation for Your Home
- How to Get the Best Interest Rates on Second-Hand Car Loans
- How to heck ISO 9001 Certificate Validity?
- How to Help Your Child Learn Financial Skills
- How to Hide Your Device’s Location
- How to Host a Bridal Party
- How to Improve JPEG Image Quality: 5 Best Ways
- How to Improve Your Employee’s Productivity?
- How to Improve Your Restaurant’s Table Turnover Rate
- How to Improve Your Sales Demo and Close Deals
- How to Increase Your NAD?
- How to Invest in Mutual Funds in Kuvera
- How to Know If Your Site Is Safe for Online Gaming?
- How to Limit Screen Time Easily on Android and iPhone with Parental Control App
- How to Liquidate a Company in Estonia?
- How to Make Patient Experience a Priority in Your Medical Practice
- How To Make Your Furniture Business Stand Out
- How to Master Your Next Medical Imaging Assignment – Secrets Revealed
- How to Move Files Between Cloud Storage – Easy & Free
- How to Optimize Your Company for Hybrid Work
- How To Organize A Cross Country Move
- How To Overcome Failure In Your Life?
- How to Plan Ahead for a Career Change
- How To Plan The Perfect Silent Disco Party
- How to Play Like a Pro? Check Out 5 Pro Tips
- How to Play Online Games Like a Pro: Tips and Tricks
- How to Play Online Games Safely and Avoid Scams
- How to Prepare Your Condo for Housewarming
- How to prevent entertainment apps from stealing your data and personal information
- How to Recover Data from Mac External Hard Drive
- How To Recover Deleted Files From an SD Card
- How to Recover Lost or Deleted Data on Windows 11?
- How to Recover RAID Data with RAID Recovery Software?
- How to Recover Vanished Video Files
- How to Redeem Your Cryptocurrency Bonus: A Comprehensive Guide
- How to Reduce Lag in Modern Warfare 2
- How To Reduce Slips, Trips, And Falls in Your Business
- How to Repair Corrupt MKV Video Files?
- How to Repair Damaged PDF Files
- How To Repair MP4 File with Best Video Repair Tool
- How to Responsibly Manage Your Company Data
- How to Safely Drive a Car in California
- How to Save Money on Your Favorite Tech Products in Australia
- How to Save Money on Your Fuel Bill
- How to Shop For Clothes on a Budget
- How to Start a Cryptocurrency Exchange
- How To Start DevOps: A Complete Guide
- How to stay healthy while travelling?
- How to Stay Top of Mind with the Right Promotional Products
- How to Stream Entertainment Games
- How to Streamline the Documentation Process for Property Loan
- How to Take Advantage of Online Game Loyalty Programs and VIP Rewards
- How to Take Care of Your Mental Health When You’re Stressed
- How to Transfer Data From iPhone to iPhone/Android Wirelessly
- How to Transfer Photos/Videos from iPhone to iPhone/Android Wirelessly
- How to Unlock iPhone without Passcode the Easy Way?
- How to Use Birth Control Like Your Life Depends on It (Because It Might)
- How to Use Color to Decorate Your Home?
- How To Win In Online Game in 9 Easy Steps
- How to Win Playing Top Online Games
- How to Write a Dissertation? Guide to Structure & Content
- How to Write an Illustration Essay Paper: Topic Ideas and Format
- How Trauma Affects An Individual 10: Things You Should Know
- How Virtual Reality is Enhancing the Online Game Experience
- How Will No-Code Impact the World for the Better?
- How You Can Bring Historical Characters Back to Life with Face Swapping in Video Online
- How Your Smartphone Can Be Your Pocket-Sized Relaxation Tool
- HubSpot Content Hub vs. Traditional CMS: Which Is Better?
- Hydrovac Excavation: The Evolution of Safe and Efficient Digging Techniques
- I Want to Work in IT, Where Do I Start?
- Igsnap: Redefining Instagram Interaction for Android Users
- Impact of Advanced Technology on Various Games Using Cryptocurrency
- Importance of Document Management Software to the Law Firm
- Importance of Mental Health Post Pandemic
- Important Areas to Include in a Tech Business
- Improve Your Soccer Gaming with Expert Tips: Soccer Gaming Tips Basics
- Increase Your Home’s Luck With These Five Tips
- India – The Oasis in the Desert for the Stock Market?
- Indiana’s Guide to Long-Term Care: Providing Quality Care for a Lifetime
- Indulge in Ultimate Luxury Shopping at One Marina Gardens Condo near Marina Bay Sands and Raffles City Shopping Centre
- Inflation Report – Possible Surprises for the US Stock Market?
- Injuries With Delayed Symptoms Following A Car Accident
- Innovative Solutions for Women’s Health: What’s New?
- Inside the World of Mobile Gaming: Insights on Players and Their Gaming Habits
- Interactive Gaming Challenges: How Missions and Rewards Change the Game
- Interesting Trends to Watch in Gaming
- Introduction to NLP Library: SpaCy Library in Python
- Introduction to Reels
- Investing in Condos with Deferred Payment Schemes (DPS)
- Investing in Condos with Lifestyle Amenities in Singapore
- iPhone Data Transfer App – FoneTool Free Download & Reviews
- Is Bitcoin Still a Viable Investment Option?
- Is It a Good Idea to Buy a Refurbished iPhone?
- Is It Ever Too Late to Get a Lawyer?
- Is Microsoft’s Ban on Crypto Mining in Cloud Services a Reason for Miners to Worry?
- Is Nanotechnology the Future of Manufacturing?
- Is SQL going to be replaced in the Future?
- Is VR the Future of Gaming?
- Is Yoga Considered a Medical Expense? Understanding Insurance Coverage
- Is Your IT Support Company Letting You Down?
- Keeping Your Home Tidy: Choosing The Right Maid Service For You
- Key Points To Double-Check In Your Two-Wheeler Insurance Document
- Know About The Different Types of Games online
- KuCoin Review – How the KuCoin Bot Can Help You Get the Most Out of Your Crypto Portfolio
- Law Firm Technical Services: What You Need to Know
- Licenses for EMI Structures in Lithuania
- Lifestyle Considerations for Future House and Condo Owners
- List of Gemstone Names by Color and Type
- Load Shedding in Cape Town and How to Find the Load Shedding Schedules
- Local Flavors in Global Arenas: How Cultural Elements Influence Indonesia’s Favorite Online Games
- Locate Parcel From All Around The World With Ordertracker
- Low-Dose Naltrexone Is Emerging as an Effective Treatment for Long Covid
- Lower Your Cell Phone Bill: Tips and Tricks
- Lucrative Social Media Jobs
- Luxurious Escapes in Udaipur: Top Hotels for a Memorable Stay
- Lyndenwoods A Prime Location for Academic Excellence at Fairfield Methodist Secondary School
- Maintaining Home Comfort: An In-depth Look at Plumbing and HVAC Services in Bellingham, WA
- Make Money with IPL Cricket
- Make These 4 Changes Now to Save Money During Inflation
- Making evidence-based and rational choices
- Making the Right Choice: Selecting a Supplement Manufacturer for Your Wellness Business
- Marketing Mavericks: Rise to Prominence in the World of Online Gaming
- Mastering Data Flow with ETL Processes
- Mastering the Art of Effective Delegation
- Mastering the Art of Selecting Your Ideal Gaming Destination
- Maximizing Brand Value: Why Every Brand Needs to Monitor MAP
- Maximizing Comfort and Convenience with Charter Bus Rental Services
- Maximizing Compensation in a Personal Injury Case
- Medical Malpractice and Personal Injury: Differences to Consider!
- Meeting Aerospace Electronics Demands with CNC Aluminum
- Memecoins Make a Comeback Despite Crypto Downturn
- Mental Health, and Substance Abuse: The Connection You Need to Know About
- Metaverse and Gaming: How VR is Creating Persistent Virtual Worlds
- Microsoft Acquires Activision Blizzard for $68.7 Billion
- Mind Games: The Psychological Benefits of Playing Video Games
- Mobile Application Development: Top Factors to Consider for Success
- Mobile Gaming – The Future of Online Games
- Modern Home Design Trends in Singapore for a Chic Look
- Modern Tech Devices to Improve Health and Well-Being
- Moissanite – the New Diamond?
- Momentum Oscillator – The Best Momentum Oscillator to Trade With
- Money Management Strategy for Low-Income Earners to Become Rich
- Mortgage Interest Rate Predictions for April 2023
- Mr. Beast Tops Forbes’ ‘YouTube Content Creator’ List for 2021
- Mulching Application Guide 101: Application Areas of Mulchers
- MuleSoft: A Comprehensive Guide to Integration Platform, Benefits and Use Cases
- Must Knows If You’re Getting Started with Workload Automation
- Must-Have Evening Picnic Essentials
- MyRecover – Windows Data Recovery Software
- Myths about Payroll Management Systems That Are Keeping You From Growing
- Navigating Legal Challenges After an Arrest
- Navigating Legal Protocols in Digital Finance
- Navigating the Cost of Restaurant Management Software Development: Market Forecast in 2024 and Key Considerations
- Navigating the UX Design Process from Research to Testing
- Navigating the Web Design Maze: A Small Business Owner’s Ultimate Guide
- NEBDN and GDC-UK: The Importance of Accreditation for Dental Nursing in the UK
- New Options in Sports Entertainment
- Next-Gen Computers: Exploring the Latest Technological Advancements
- Nissan Going Greener – What Is the Company Up To?
- Normal Charges For Assault and Child Abuse In Pennsylvania
- Norwood Grand Woodlands Gateway to Convenience and Connectivity on Woodlands Avenue 2 and 5
- Nosebleeds and Their Causes and Treatments
- Novice-Friendly Games: How to Find them Online?
- Nutrition For Feline Friends: Choosing The Best Diet For Your Beloved Cat
- Online gaming: The place where dreams turn into reality
- Online Scammers Fake Connection With Central Bank of Ireland to Find New Victims
- Online Therapy Providers That Take Insurance in 2023
- Online Trading
- Open-Source Solutions for a Multi-vendor Marketplace
- Optimizing Google Ads Payments: A Guide to Selecting the Right Payment Cards
- Outstanding Entertainment And Gaming Platforms In Africa
- Personal Injury Claims: An Overview Of Different types
- Picking Between Dedicated Servers and VPS at USA Datacenter
- PicWish: The Best AI Photo Enhancer and Editor
- Planning Your Diet And Nutrition: Stay Away From These 9 Myths
- Plesk Control Panel: Simplifying Web Hosting on VPS for Windows and Linux
- Pre Workout Food: What Should I Eat Before Workout
- Preventing the 4 Major Multi-Cloud Security Challenges
- Procedures for Payroll in Small Businesses
- Prominent Game Testing Trends that Will Likely Shape the Coming Years
- PropTech and Energy Management as Business Catalysts
- Pros and Cons of Multitasking While Working From Home
- Protect your AI Infrastructure with a DSPM Tool
- Proven Benefits of Hiring a Social Media Agency
- Psychometrics of Play: Understanding Player Behavior in Online Gaming
- Pull Up Banners
- Puzzle Solving and Adventure: The Magic Formula of Treasure Hunt Games
- Python Array: What Is It?
- Python vs Java: Differences that Developers Should Know
- Quantitative Finance: A Deep Dive into Algorithmic Trading
- Questions to Boost Remote Employees’ Productivity
- Quick and Easy Fix: Replacing the Missing Knob on Your Samsung Range
- Quick Home Repairs You Can Tackle Yourself in Singapore Without Breaking the Bank
- Quill Forms VS Gravity Forms
- Reaping Big Rewards with Crypto Gaming
- Reasons Why You Should Trust a Personal Injury Attorney
- Rebalancing Your Portfolio? Make Sure to Avoid These Mistakes!
- Recession Fears – Is the Stock Market Crash Inevitable?
- Reclaim Your Health with Heart Checkup and Vitamin B12 Testing in Mumbai
- Recover Deleted Files in Windows 10 With/Without Software
- Recovering and Healing After a Motorcycle Accident
- Recruitment Efforts with the Top-Rated Free CRM
- Reliable VMware Backups and Rapid Restores
- Remote Control PC from iPhone with AnyViewer
- Residential Proxies improve YouTube experience
- Restoring Windows to Previous Date [Windows 11/10]
- Revitalize Your Mind and Body with Yoga in Singapore
- Revolutionizing Business Operations with Digital Forms
- Role of Influencer Marketing Agencies in UAE for Driving Sales
- Safe Ways of Cashing out From Online Games in India
- Safer Aircraft via ARP4761A
- Safety and Security Tips for Online Games Players
- Safety Measures You Can Take for Your Property
- Sales Coaching Training will benefit your business
- Sales Training Programs: The Best Business Investment a Firm Can Make
- Same Day Weed Delivery
- Samsung Adopts AMD GPUs For Its Latest Mobile Devices
- Satin Vs Silk Pillowcase: Which Material Is Better for Sleep?
- Saudi Arabia Gears Up to Host the 2024 Esports World Cup
- Save2be: Pioneering Seamless and Effortless YouTube Video Downloads
- Scale Up Your Business With The Right KPIs
- Science behind HBOT and its Health benefits beyond the chambers
- Screen Recording Software Comparison: Finding the Right Solution for Your PC
- Seaside Splendor: Exploring Italy’s Diverse Beachfront Luxury Real Estate
- Seasonal HVAC Maintenance: Navigating Indianapolis’ Extreme Weather with Ease and Efficiency
- Secrets to Moving House Quickly Using Technology
- Secure File Hosting: How to Keep Your Data Protected in 2025
- Securing Online Communication with Microsoft Teams
- Securing Your Gameplay: How Crypto Ensures Safety and Transparency
- Securing Your SaaS Landscape: A Multi-Layered Defense Against AI Risks
- Selling Your Home In Brampton How To Get The Best Price For Your Property When You’re Planning To Move
- SEO Timeframes – Understanding the Factors that Influence Results
- Should Apartment Marketers Use Chatbots On Their Websites?
- Should You Consider a Truck Dispatcher Course?
- Should You Get an iOS or Android Phone for Gaming?
- Should you include branding with your corporate gifts?
- Sihoo DORO C300: The Best Office Chair for Computer Work – Enhance Productivity
- Simple Steps to Recover Unplayable and Inaccessible Videos
- Singapore Home Makeovers – Expert Ideas to Refresh and Update Your Space
- Singapore Real Estate 2024: Emerging Trends to Watch for Savvy Investors
- Singapore’s Best Neighborhoods for Young Couples Starting a Family
- Singapore’s Most Popular Home Renovation Design Styles Revealed
- Single Sofas: Compact, Versatile, and Stylish
- Sizzling Seven’s Showdown: Mastering Triple 7 Treasures
- Skye Holland Enhancing Connectivity through the URA Master Plan’s Transport Network Improvements
- Smart Hacks for Quick Home Repairs Around Your Singapore Home
- Smart Heating and Cooling Systems
- Smart Living: How Condo Residents Can Benefit from Mobile Apps
- Smart Strategies to Boost Your SERP Rankings
- Smart Strategies to Refinance Your Car Loan for Better Savings
- Social Surge: The Ultimate Guide to Buying Instagram Followers from Reputable Sites
- Soft Skills That Characterize an Outstanding Security Professional
- Solana Volume Bot: A New Force in Blockchain Trading
- Some most important things you can do to protect yourself and your gains
- Some Stress Management Tips for Students
- Sorare, MLB NFTs: Everything You Must Know
- Space-Saving Furniture Ideas for Singapore Apartments
- Spacious and Stylish: Open-Concept Renovation Ideas in Singapore
- Speed and Security: Why VPS is Essential for Hosting Large Files
- Speeding Up Response Times in the SOC with AI
- Splunk: The All-in-One Solution for Big Data Analytics
- Staying Ahead of the Game: Mobile Gaming Challenges, Trends, and Opportunities in 2022
- Staying Comfortable for Long Gaming Sessions
- Staying in the Know: A Look at the Top Gaming Trends for 2023
- Strapi vs Traditional CMS: What’s the Difference?
- Strategies for Increasing Employee Engagement with WFM Solutions
- Streamlining Your Bitcoin Mining Operations with Miner Management Software
- Strengthening digital trust as the foundation for digital security
- Stylish and Functional Home Renovation Ideas for Singaporean Apartments
- Substance To Social MediaL: Here Are 9 Things That People Are Addicted To In 2023
- Sustainability Steps Businesses can take Today for a Greener Tomorrow
- Sustainable Living Room Makeover Ideas for Eco-Conscious Singapore Homeowners
- Sustainable Transportation Initiatives: Bike Lanes and Walkability in Singapore
- Tailored Websites For Tech: Design Principles Every Msp Should Implement
- Take Control of Your VPS: Why Control Panels are an Indispensable Tool
- Takeaway Tips To Making The Most Of Your Collaboration With Android App Development Company
- Tapping Into the Future: Key Trends and Predictions in Mobile Gaming
- Teamwork and Tactics: Strategies for Success in Multiplayer Treasure Hunt Games
- Tech Savvy Tips for Better Health
- Tech That Changed Money Forever
- Technology Solutions to Emergency Situations
- TED Talks That Anyone Working in List Open Ports on Linux Should Watch
- Thanksgiving 2022: Which Stores Closed On Thursday
- The 4 Features of Enterprise Secure File Sharing
- The 4 Technologies That Make Herbal Supplements Possible
- The A-Z of Home Renovations in Singapore – Comprehensive Tips and Inspiration
- The Advantages Of Aluminium Extrusion Manufacturing In China
- The Advantages of Outside Financial Advice
- The Anatomy of a Data Breach: From Attack Vectors to Remediation
- The Art of Anonymity: How Proxy VPNs Protect Your Digital Identity
- The Art of Caring: A Comprehensive Guide to Pediatric Orthopedic Care
- The Art of Spinning: Mastering Game Online for Fun and Profit
- The Benefits Of Copper Workstation Sinks
- The Benefits of Data Mesh Technology for Businesses
- The Benefits of Outsourcing IT Services
- The benefits of playing online games
- The Benefits of Reading Manga in Toonily: A Guide to Help You Fall In Love With Comics Again!
- The Benefits Of Using An Updated Facility Management Software
- The Benefits of Using FamiSafe to Track Cell Phone Locations
- The Benefits: A Comprehensive Guide to Premier Vision
- The Best Lipgloss for Summer
- The Best Vitamins To Add to Your Daily Regimen
- The Best Way to Level Up in League of Legends: Looking for Gamers
- The Business of Soccer in Mexico: Economics and Sponsorships
- The Business Value of Good App Design
- The Complete Guide for Commercial Real Estate
- The Complete Guide to Business Telemarketing Services
- The Convenience and Comfort of a Foldable Floor Mattress
- The Critical Role of Electronic Logging Devices (ELDs) in Truck Accident Cases
- The Crucial Role of an OBGYN Expert Witness in Medical Legal Cases
- The Difference Between Conversion APIs and Pixels
- The Different Types of Debt Instruments
- The Easy Fix for iPhone Error 4013 using the Best Software for Mac and PC
- The Engine Behind the Avatar: Exploring Game Development Technologies
- The Esports Explosion: An Analysis of Its Meteoric Rise in the Business World
- The Evolution of Cyber Threats: How Data Detection and Response Keeps Pace
- The Future of AI Automation: Comparing GPT-4.5 API and Gemini 2.5 API for Workflow Optimization
- The Future of Augmented Reality: How AR Enhances the Treasure Hunt Gaming Experience
- The Future of Automotive Technology: Impact on Insurance and Insurers
- The Future of Fax: Exploring Innovation in iPhone Apps
- The Future of Flavour: Why Prefilled Pods Are Taking Over in 2025
- The Future of Money: What to Expect from the World of Finance in the Coming Years
- The Future Of NFTs
- The Future of Online Gaming: Exploring Emerging Technologies
- The Game-Changing Role of Cloud-Based Data Storage Solutions
- The Great App Debate: Progressive Web Apps vs. Native Apps
- The Hidden Risks of Ignoring Leaky Faucets
- The History of Game Machines: From One-Armed Bandits to Online Games
- The History of Tapestries
- The Impact of Economic Indicators on Stock and Share Results
- The Impact of Interest Rates on Singapore Home Loans
- The Impact of Music on Online Game Engagement
- The Impact of Social Emotional Learning (SEL) in Schools: Fostering Emotional Intelligence and Well-being
- The Impact of Website Speed on User Experience and SEO
- The Impact that Street Fighter II Has Had on the Fighting Game Genre
- The Importance of a Durable Solar Panel: Choosing Jackery for Long-lasting Power
- The importance of building a reliable data center infrastructure for business
- The Importance of Good Commercial Mold Removal
- The Importance of Mobile-Responsive Design
- The Importance of Security Features in Condo Investments
- The Importance of Seed Keywords and Keyword Intent in Ecommerce SEO
- The Importance of Using a VPN While Gaming & Watching Your Favorite Sporting Events
- The Increasing Impact of Social Media on News Consumption
- The Joy of Collecting Ancient Art: Embracing Pre-Columbian Masterpieces for Home and Office
- The Key Components of Internal Developer Platforms
- The Latest Technology Trends in Sweden for Norwegian Gaming Sites
- The Most Advanced Technologies Used By Online Businesses Nowadays To Protect And Store Sensitive Data
- The most Effective Technique to Correctly Utilize Low Tack Transfer Tape for Plastic
- The most notable benefits of social media marketing according to an agency in Budapest
- The Most Promising Wearable Medical Devices to Invest In Today
- The Most Recommended Android App to Transfer Data Between Devices
- The Path to Becoming a CEO
- The Path to IT Support Excellence: Powerful Strategies for New Professionals
- The Perfect Mummy Makeover Clinic in Turkey: Tips and Things to know
- The Power of a Dedicated Server: A Software Developer’s Dream Machine
- The Power of Entertainment: Creating Relaxation and Connection in a Fast-Paced World
- The Power of Mindfulness Meditation
- The Power of Positive Thinking in Mental Health
- The Power of UiPath_ Revolutionizing Automation
- The power of visual communication: How data visualization techniques enhance business insights
- The Pros and Cons of Conducting Exit Interviews
- The Pros and Cons of New Developments vs. Resale Properties in Singapore
- The Prospects of Play-to-Earn NFT Game Development in the Current Crypto Scene!
- The Quick and Easy Ways to Transfer Data from Samsung to the iPhone 15
- The Real Estate Market in Charles County, MD: A Study in Adaptation
- The Redmi K50 Series Could Rival iPhone’s Haptics Technology
- The Relationship Between Nutrition And Fitness: An Expert Analysis
- The reliable Joseph-Antoine Bell
- The Rise of Contactless Payment Apps in Small Businesses
- The Rise of Customisable iPads: Will Modular Design Be the Next Big Thing?
- The Rise of Smart Cities: Singapore’s Vision for Urban Innovation
- The Rise of Smart Condos: How Technology Is Enhancing Resident Experience
- The Role of Artificial Intelligence in Online Gaming Design
- The Role of Carpets in our Home
- The Role of Credit Score in Getting a Business Loan Online
- The Role of Custom Software Solutions in Transforming Cloud Storage
- The Role of Family Nurse Practitioners in Post-COVID Healthcare
- The Role of Milkplan Cooling Tanks in Modern Dairy Farming
- The role of real-time monitoring software in reducing call center AHT
- The Role of Technology in Nobul’s Real Estate Approach
- The Science Behind Arabian Perfumery: Chemistry and Art Combined
- The science behind personality and the role of mental health counselors
- The Secret to Adding More Excitement to Any Sports
- The Sen Condo’s Perfect Location Journey Across Singapore with Ease via the Pan Island Expressway (PIE)
- The situation in Sri Lanka after President Resigns
- The Technological Evolution of Online Gaming
- The Technology Behind Sportsbooks
- The Three Solutions that Swallowed DLP
- The Top European Cloud Storage Solutions
- The Trend Toward Urgent Care in Medical Real Estate
- The True Cost of Having a Family
- The Ultimate Guide for Fishing Reels
- The Ultimate Guide to Creating Compelling Audiobooks with AI Voice Generator
- The Ultimate Guide to Hunting Clothes: Choosing the Right Gear for Success
- The Ultimate Guide to Living Room Makeover Ideas for Singaporean Families
- The Ultimate Guide to Paper Bags, Parcel Bags, Postage Bags, and Mailing Bags with Mr Bags: Practical, Eco-Friendly, and Loved by All
- The Ultimate Guide to Spotting the Best Online Games
- The Ultimate Guide to UPI BHIM App Download and Setup
- The Ultimate Guide to Wall Paint: Trends and Tips for 2025
- The Use of AI in Search Engine Optimization
- The Use of Cryptography in Online Games: Ensuring Integrity and Fairness
- The UX Design Process – From Research to Testing
- Things To Ask Your Surgeon During a Rhinoplasty Consultation
- Things to Check While Buying a Used iPhone
- Things to Consider Before Selecting a B2B eCommerce Software
- Things to Consider in the Process of Addiction Recovery
- Things to Consider When Hiring a Truck Accident Attorney
- Things to Think About Before Hiring a Car Accident Lawyer
- Timeless Modern Home Design Trends in Singapore for a Chic and Stylish Look
- Tips for a Hassle-Free Online Games Installation: Your Complete Guide
- Tips for a Smooth Website Migration: Moving Your Site to a New Host
- Tips for Creating a Data Loss Prevention Policy
- Tips for Creating and Editing Entertaining Videos using Movie Maker
- Tips for Identifying Premium Games Online
- Tips For Learning How To Trade The Markets
- Tips for Men Looking to Learn New Hobbies and Skills
- Tips for successful trading
- Tips for Using Digital Marketing Tools To Improve Your SEO
- Tips on How to Keep Healthy for Working Parents
- Tips to Ease the Stress of Moving For Your Pet
- Tips to Flirt With a Married Woman Effectively and Get More Dates
- Tips to Maintain Your Credit Balance
- Tips to Transform Your Condo Space into a Tranquil Oasis
- Top 10 Finnish Entertainment news outlets
- Top 3 Best Web3 Gaming Platforms to Check Out
- Top 4 Approaches to Quantitative Research in Business
- Top 4 Ways to Fake GPS Location on iPhone Safely and Efficiently
- TOP 5 CDN Services for Game Apps with Global Audiences in 2023
- Top 5 Classic Games Variations to Try Out
- Top 5 Cyber Sports Trends
- Top 5 Ecommerce SEO Strategies for Boosting Your Online Sales
- Top 5 Iconic Games to Play
- Top 5 RPG Video Games to Play in 2023
- Top 5 Skills You Will Learn When Becoming a Real Estate Agent
- Top 5 Tips for Starting a Healthcare Business and Making It Grow
- Top 6 Global HR Practices for 2022
- Top 6 Online AI Video Translator Review in 2023
- Top 6 Perks of Web Designing Services in Website Optimization
- Top 7 AI Apps to Boost Business and Productivity
- Top 7 iOS System Repair software in 2022 (Windows and Mac)
- Top 8 Pet-Friendly Communities in Dubai
- Top API Protection Tools
- Top Benefits of Choosing an LG Double Door Fridge
- Top Benefits of Hiring a Product Liability Attorney for Faulty Products
- Top Benefits of Outsourcing Link Building to Virtual Assistants
- Top Excel Consultants: How to Find the Right Expert for Your Business
- Top Features of an Online Share Trading App to Analyze Idea Share Price Easily
- Top Influencer Marketing Agency for Top-notch Marketing Services
- Top Influencer Marketing Agency in the UAE 2024
- Top Neighborhoods for Real Estate Investment in Singapore
- Top Off-Page SEO Trends That You Need To Check Out
- Top Online Bootcamps for UI/UX in 2022
- Top Reasons to Say Yes to Royal TV for Sports Fans
- Top Secrets to Channeling Positivity in Your Life
- Top Software Testing Certifications for 2024
- Top Software Testing YT Channels for 2024
- Top Tools to Consider for Docker Development
- Top Trends in Crypto Gaming
- Top UGC Agency in India
- Top Video Production Company In India
- Top Video Production Company in India
- Top VPN Services for Enhanced Privacy and Security
- Top Way to Sync Google Photos to Google Drive
- Trading 101: A Beginner’s Guide to Mastering the Basics
- Transform Your Home with Innovative Storage Ideas in Singapore
- Transform Your YouTube Channel: The Ultimate Guide to Using Filmora’s AI Thumbnail Creator!
- Transformative Impact of the URA’s Vision for Parktown Residences Tampines on Quality of Life and Investment
- Transforming Senior Living: Essential Housing Solutions for Singapore’s Ageing Population
- Travel insurance benefits for families: Why it’s essential for travelling with children
- Traveling Light in Philadelphia
- Trenchless Pipe Repair: A Cost-effective and Efficient Solution for Plumbing Issues
- True or Fake AI Data Repair Tool?
- Try Your Luck – Winning Strategy
- Twill: The Best News Payment Platform for Businesses
- Types of Barcodes Scanners& Reasons to Acquire a Holster
- Types of Car Insurance Plans you Should know About
- Types of Social Engineering Attacks in 2022
- Ultimate Guide: How to Save Big in College — 25 Smart Strategies
- Ultrasonic Flow Meter: What Is It? How Does It Work?
- Uncover the Secrets of Green Herb: A Deep Dive into the Best Flavours and Top Brands
- Uncover the Secrets of Headhunters in New Orleans
- Understanding How Online Bookmakers Set Odds in 2023
- Understanding IR Sensors: A Comprehensive Guide
- Understanding Mixed Hearing Loss: Causes, Symptoms, and Treatment Options
- Understanding Private Student Loans: What You Need to Know
- Understanding SAAS Boilerplates And How They Can Help
- Understanding Search Algorithms Curated By Tech Enthusiasts
- Understanding Solar Hot Water Systems: A Deep Dive into Their Components and Functionality
- Understanding the Different Types of Games Available in Austrian Market
- Understanding the Role of Viasox Compression Socks in Diabetes Care
- Understanding Wildcard SSL certificates: What they are and how they work?
- Unlock Agile Marketing with Ad Hoc Reporting
- Unlock New Strength with S23 SARM and Stenabolic
- Unlock the Full Potential of Office 365: Proven Tips and Tricks
- Unlocking Potential: Strategic Implementation of Dental Software for DSOs’ Long-Term Success
- Unlocking the Potential of 3D Printing Services with the 3DEXPERIENCE Marketplace
- Unlocking the Potential of Mempool Space: A Comprehensive Guide
- Unlocking the Potential: The Science Behind Weight Loss Medication
- Unlocking the Secrets of Timeless Online Games Popularity
- Unlocking Your GPS: Top Location Spoofer Apps for 2023
- Unplugging Activities to Try at Home
- Unveiling Advanced Marketing Tactics: The Online Game Approach
- Unveiling Iran: The Ideal Destination for Affordable Rhinoplasty
- Unveiling Plesk Web Pro Edition: Empowering Web Hosting Management
- Unveiling the Power of Investment: Why Your Website Needs Premium Server Hosting
- Upgrade Your Home Effortlessly with the Bajaj Finserv EMI Network Card
- Useful Apps to know about if you’re Thinking about Rebooting Your life
- User Research Tips, Insights, Methods, Benefits & Tools
- Using OnlineSim for SMS Verification Codes
- Valorant Ban Waves – From Detection to Ejection
- Valuable Tips to Help You Select a Good Spy App
- Valyuz Review – an Alternative Banking Solution that Makes Mass Payments Simple
- Video Production in San Francisco Made Easy
- Virbo vs Elai: AI Avatar Battle – Features, Pricing, and Performance
- Virtual Assistants in Improving Healthcare Workflow
- VoIP Caller ID and Other Features
- Volcán Tajumulco: Active Volcano
- Wall Mounted vs Floor Standing Rack Cabinets
- Warzone Cheats Everything you need to know
- Watch and Win: Unlocking the Secrets of Online Gaming Bonuses
- Ways to Reduce Your Monthly Expenses
- Ways to Stay Safe While Playing your Favourite Video Game
- Ways You Can Violate A Restraining Order
- Weathering the Waves: The Durability and Versatility of Custom Floating Dock Solutions
- What Accessories Should You Add to Your Business Wardrobe?
- What are Industrial Lifting Bags: features of choice
- What Are Temporary Buildings?
- What are the Advantages of Hiring a Property Manager?
- What are the Best Ways of Empowering Employees?
- What Are the Common Challenges Faced by Family Caregivers?
- What are the Features of Pendo Alternatives?
- What Are the Key Features of Call Center Software?
- What Are the Legal Options for Hair Relaxer Victims? A Lawyer’s Perspective
- What are the Online Gaming Business Opportunities Available for Entrepreneurs?
- What Do You Need For IPTV Video Streaming?
- What Does a Well Woman and Well Man Blood Test Check For?
- What Does CFS Mean On Instagram
- What Does the First Half of 2023 Look Like for the Stock Market?
- What Happens When We Die According to Religious Beliefs?
- What innovations have recently taken place in the world of web hosting?
- What is a Gaming PR Agency and why do gaming businesses need them?
- What is a PAT, Aka Portable Appliance Tester?
- What Is a Smart Home and Why You Need to Invest in One?
- What Is a Subdomain and How Does It Impact SEO
- What is Antivirus Software?
- What Is Aviator Crash Game And How To Play It?
- What is Content on Websites? Why You Need Moderation
- What Is Customer Identity and Access Management, and How Can It Protect Your Business?
- What is Document Generation?
- What is E-commerce Web Design Agency London? Why should E-Commerce businessmen pay importance to this?
- What Is Fleet Maintenance? 4 Benefits of Fleet Maintenance
- What Is Futures Market Making?
- What is HR Technology and How It Can Help Your Company Achieve More
- What is MLOps and why is it important in today’s world of data-driven decision making
- What Is Schizophrenia And How Does It Affect Individiuals: A Guide By Experts
- What is Sleep Divorce and How Can it Help You Sleep Better?
- What Is Tezos Blockchain Network – Should You Invest in It?
- What is the Meaning of Super Top-Up Health Insurance – Everything You Need to Know
- What is the most effective treatment for Eczema?
- What Makes a Gift Memorable? 5 Key Factors to Consider
- What people can cook with what they already have in the fridge?
- What Should You Do After Having Breast Augmentation?
- What Should You Know Before Exploring Original Pukhraj Stone Jewelry
- What Skills are Required for C++ Engineer?
- What to Do in Case of Drunk Driving Charges
- What to Do When Filing a Los Angeles Personal Injury Lawsuit
- What to Expect during your Clinical Placement, and how to get the Most out of it
- What to Expect When Working with a Travel Nurse Agency
- What to Know About Contextualized Data
- What To Look For When Buying A Refurbished Phone
- What to Look for When Renting a Commercial Warehouse
- What to Play Online When I Am Stuck at Home
- What You Need to Know Before Choosing Your Website Host
- What You Need to Start Selling on Amazon FBA
- What’s new in Cloud Storage? A look at the Latest Offerings from Cloud Storage Providers
- When Should a Small Business Consider Outsourcing?
- When Should You Provide Your Phone Number to Websites?
- When to Use the Word Pendejo
- When you need EV Code Signing Certificate
- When Your Home Needs More Than a Roofing Patch
- Where Should Banjo & Kazooie Go Next?
- Where To Find the Best Overboard Rescue Equipment
- Which App is Considered Essential for Every Cricket Enthusiast?
- Which Coffee Printer Suits me?
- Why 73% of Enterprises Choose Atlassian Consultants Like Evelon in 2025
- Why a Massive Following on Facebook is Essential
- Why a Secured Loan Could Be Better Than Remortgaging
- Why A2 Ghee Is the Healthiest Choice for Your Kitchen
- Why Accounting Software is Essential for Comprehensive Business Management in India
- Why an Enterprise Virtual Data Room Is Essential for Your Business
- Why an interior designer would recommend kitchen splashbacks
- Why Are Online Games Gaining Popularity Today?
- Why Are Video Games So Popular and How to Play Them
- Why can’t Retail Businesses Survive without Address Verification Services?
- Why Custom Web Apps are Good for E-commerce Businesses
- Why Data and Call Center Analysis is Crucial for Your Customer Services
- Why do companies need an executive sales recruitment agency?
- Why Do Custom Packaging Boxes Matter For Your Business?
- Why Do People Love Intense Action Movies?
- Why do small businesses need accountants?
- Why do you need regression testing & how to successfully perform it?
- Why does Hulu have so many Ads Even though I Pay for my Subscription?
- Why Every Agency Must Update About SEO Latest Techniques?
- Why Holistic Healing Works in Teen Rehab
- Why is Instagram promotion necessary for your business?
- Why is SEO Important for your Business?
- Why Is Visual Design Important Everywhere?
- Why Listing on the Top Online Business Directory is a Must-Have for Your Business
- Why Managing Your Cryptocurrency Portfolio Is The Only Skill You Really Need
- Why Negative Reviews Help Your Online Business
- Why Open an Online Savings Account?
- Why Personalization Is Key To Selling Insurance In 2023
- Why Professional EV Charger Installation is Crucial for Safety and Longevity
- Why React Native is the Go-To Choice for Startup Mobile App Development
- Why Reviews Are Important: A Gamer’s Perspective
- Why Should You Focus On Witness Statements And Testimonies After A Car Accident In Toledo?
- Why Should You Start Your Very Own Coffee Business?
- Why Simulcast Is The Best Way To Expand Your Reach
- Why We Need An Auto Shipping Expert
- Why you should consider a crypto tracker app
- Why You Should Consider Defined Benefit Pension Plan
- Why You Should Consult A Lawyer Following A Truck Accident?
- Why You Should Hire an Accident Attorney
- Why You Should Host Your Website on A Cloud Server and Not on an ordinary One
- Why You Should Insulate Your Garage
- Why You Should Order Items in Bulk
- Why You Should Play Fantasy Cricket?
- Why You Should Use Pixlr to Optimize AI Artwork
- Why Your Smartphone is the Ultimate Online Gaming Companion
- WIFI PTZ Battery + Solar Camera
- Will the Ongoing Cardano Improvements Have an Effect on its Price?
- Windows Server in the Cloud: Microsoft Azure Vs Amazon Web Services
- Windows Server Management: Tools and Techniques for Efficient Administration
- Winning Strategies For Online Games
- Winter-Proof Your Home: Your Essential Guide to Heating System Maintenance and Efficiency
- With A Burgeoning Turnpike System Retail Real Estate in Florida Getting a Big Boost
- With online games, you may increase your passive income
- Women’s Rise in the Game of Golf
- Woolash, The Best Eyelash Serum I’ve Ever Bought!
- Your Complete Guide to Multi-Functional Storage Solutions in Singapore
- Your Guide to Top PG Programs in Digital Marketing
- Your Ultimate Guide to GPS Tracking for Fleet Vehicles
- Youtube Mp3 Downloader Online For Mac
- YouTube’s Original Content Head Exec to Exit in March
- ZTE Redefines Home Entertainment with 4K DVB Zapper Set Top Box
Science / Health
- 10 Reason You Should Never Attempt To Detox At Home For An Addiction Problem
- 4 Tips for Navigating Menopause
- 4 Ways to Increase Your Vitamin Intake
- 5 Clear Warning Signs that You May Need a Root Canal
- 5 Home And Natural Remedies For Your Toothache Pain
- 5 Ways To Find The Right Buyer For Your Dental Practice
- 5 Ways to Prepare for a Sick Day at Home
- 6 Possible Signs You May be Heading for Dentures
- 7 Health Products That Help to Improve Your Overall Well-Being
- 7 Proven Strategies for Growing Your Veterinary Practice (2024)
- 7 Reasons Why Poor Mental Health Is The Leading Cause Of Addiction
- 8 Easy Steps To Get To Know Your Natural Weight Loss System
- A Guide to Shopping for Health Products When You’re a Newbie
- All You Need To Know About Air Tack Mats
- Are Peptides the Same as Proteins?
- Autoclave for hospital equipment – What should I know before buying one?
- Battling Substance Abuse And Addiction In Las Vegas: 10 Things You Should Do
- Benefits Of Velocity-Based Training For Athletes
- Best Health Care Plans- What to Look for and the Various Types
- Beyond the Aesthetics: Rhinoplasty’s Side Effects
- Can Taking THC O Gummies Strengthen Your Immunity?
- Choosing A Clinic To Treat Mental Health Issues: 7 Things To Know
- Combining sports and cardio for a dynamic workout routine
- Conjuctivochalasis: Wrinkled Eyeballs
- Contraceptive Options: Finding the Right Fit for You
- Danger of Hookah Pens
- Dental Implants vs. Traditional Dentures: Which One is Right for You?
- Detection of Phenibut in a Commercially General Product in Richmond, VA: A Topic Research
- Different Types Of ONYC Hair Clip Ins To Try
- Essential Insights into Children’s Healthcare Orthopedics: The Ultimate Guide
- Essential Insights into Milk Processing Equipment for Modern Dairy Operations
- Exploring New Advancements in Alzheimer’s Disease Treatment
- Facelift Surgery Trends You Need to Know
- Finding the Right Endometriosis Specialist in Portland: Your Comprehensive Guide
- Fluoride 101: Facts, Myths, and Common Misconceptions
- Focus on Amnesia
- From Brain to Heart: How Dubai Hospitals Offer Comprehensive Care
- Future Trends in Dentistry and How an Online Dental Nurse Course Can Prepare You
- Great Ways to Stay Fit and Healthy
- Healing from Narcissistic Abuse: Reclaiming Your Inner Strength and Raising Your Self-Worth
- Health Benefits Of Using Custom Air Filters
- Health Innovation as Part of a Benefits Strategy
- Healthier Living Through Filtration: Identifying the Premier Choice
- How DBT & CBT Therapy Helps with PTSD Treatment
- How Does the Anti-THC Spray Guarantee your Peace of Mind?
- How Hunting Can Improve Mental Health and Foster Personal Development
- How Noble’s Innovations in Medication Delivery Are Revolutionizing Patient Care
- How Often Should I Renew My CPR Certification?
- How to Choose the Right Yoga Mat?
- How To Elevate Mouth Corners
- How to Make Patient Experience a Priority in Your Medical Practice
- How to stay healthy while travelling?
- How to Take Care of Your Mental Health When You’re Stressed
- How Trauma Affects An Individual 10: Things You Should Know
- Importance of Mental Health Post Pandemic
- Innovative Solutions for Women’s Health: What’s New?
- Low-Dose Naltrexone Is Emerging as an Effective Treatment for Long Covid
- Making the Right Choice: Selecting a Supplement Manufacturer for Your Wellness Business
- Mental Health, and Substance Abuse: The Connection You Need to Know About
- Modern Tech Devices to Improve Health and Well-Being
- NEBDN and GDC-UK: The Importance of Accreditation for Dental Nursing in the UK
- Nosebleeds and Their Causes and Treatments
- Nutrition For Feline Friends: Choosing The Best Diet For Your Beloved Cat
- Online Therapy Providers That Take Insurance in 2023
- Personal Injury Claims: An Overview Of Different types
- Pre Workout Food: What Should I Eat Before Workout
- Reclaim Your Health with Heart Checkup and Vitamin B12 Testing in Mumbai
- Revitalize Your Mind and Body with Yoga in Singapore
- Satin Vs Silk Pillowcase: Which Material Is Better for Sleep?
- Science behind HBOT and its Health benefits beyond the chambers
- Some Stress Management Tips for Students
- Strapi vs Traditional CMS: What’s the Difference?
- The 4 Technologies That Make Herbal Supplements Possible
- The Art of Caring: A Comprehensive Guide to Pediatric Orthopedic Care
- The Benefits: A Comprehensive Guide to Premier Vision
- The Best Lipgloss for Summer
- The Best Vitamins To Add to Your Daily Regimen
- The Crucial Role of an OBGYN Expert Witness in Medical Legal Cases
- The Perfect Mummy Makeover Clinic in Turkey: Tips and Things to know
- The Power of Positive Thinking in Mental Health
- The Relationship Between Nutrition And Fitness: An Expert Analysis
- The Role of Family Nurse Practitioners in Post-COVID Healthcare
- The science behind personality and the role of mental health counselors
- The Trend Toward Urgent Care in Medical Real Estate
- Things To Ask Your Surgeon During a Rhinoplasty Consultation
- Tips on How to Keep Healthy for Working Parents
- Top Secrets to Channeling Positivity in Your Life
- Understanding Mixed Hearing Loss: Causes, Symptoms, and Treatment Options
- Understanding the Role of Viasox Compression Socks in Diabetes Care
- Unlocking the Potential: The Science Behind Weight Loss Medication
- What Does a Well Woman and Well Man Blood Test Check For?
- What Is Schizophrenia And How Does It Affect Individiuals: A Guide By Experts
- What is Sleep Divorce and How Can it Help You Sleep Better?
- What is the Meaning of Super Top-Up Health Insurance – Everything You Need to Know
- What is the most effective treatment for Eczema?
- What Should You Do After Having Breast Augmentation?
- What to Expect during your Clinical Placement, and how to get the Most out of it
- What to Expect When Working with a Travel Nurse Agency
- Why A2 Ghee Is the Healthiest Choice for Your Kitchen
- Why Holistic Healing Works in Teen Rehab
Technology
- Build Your Dream Website in Minutes: The Power of AI Website Builders
- How to Unformat an SD Card on Computer?
- [2023 Best Picks] 6 Voice Changers for Discord on Mac
- The 4 Different PC Cases and a Brief Guide on What to Buy
- Exploring the New Frontier of Design and Technology with Sarah Zaheer
- How Consumers Can Leverage Big Data
- How to Scale Your Software Development Team with Team Augmentation?
- SEO Analytics: Tracking Your Results
- 10 Best Mobile Application Testing Tools in 2023
- 10 Best Practices for eCommerce Website Security
- 3 Tech Startup Ideas to Look into
- 3 Ways HVAC Software Can Improve Your Productivity
- 3 Ways to Enter the IT Market
- 4 Best Ever Applications to Hide your Photos & Videos
- 4 Essential Things You Can Do Online After Moving House
- 4 Key Benefits of Hosting Quickbooks On the Cloud
- 4 Methods: How to Unlock Android Phone Without Password
- 4 Tips to Help You Transition from ADSL Broadband; It Could Be Coming to an End
- 4 Top Sustainable Practices in Geotechnical Engineering Worth Implementing
- 4 Ways QR codes are used in Marketing
- 4 Ways to Protect Borrowers’ Personal Information
- 5 Advantages of the Internet for Businesses and Students
- 5 Applications of Peptides in Industrial Biotechnology
- 5 apps that can compromise your privacy big time
- 5 benefits of Cloud Software for Medical Practices
- 5 Benefits Of Data Analytics With Modern Tools
- 5 Best Low-Investment Business Ideas You Can Start Online
- 5 Best Practices for Affiliate Email Marketing Campaigns
- 5 Easy Tech Tips to Secure Your Home
- 5 Guaranteed Ways To Streamline Remote Hiring for Recruiters
- 5 Lucrative Online Jobs That Can Skyrocket Your Income
- 5 Reasons Why You Should Use a VPN for Online Security
- 5 Tech Devices for Cleaning Your House Efficiently
- 5 Tips on Setting Up Your Condo’s Wi-Fi Network
- 5 Tips to Create a Smooth-flowing Chart
- 5 Useful Things Local SEO Experts Can Do for Your Company
- 6 Content Marketing Ideas for Small Businesses
- 6 Must-Have Home Appliances in 2024
- 6 NFTs that Run on the Solana Blockchain
- 6 Personal Tech to Stay Cool and Comfortable
- 6 Things to look for when selecting the right HTML5 Web-to-Print Software
- 6 Tips to Follow for Vue js Deployment Practices
- 7 Best SEO Tools According to Expert SEO Strategists
- 7 Must-Have Gadget Accessories
- 7 Reasons to Choose a Smart Thermostat
- 7 Ways to Speed Up Your Old iPhone In Under 5 Minutes
- 8 Benefits of Automating Manual Hospital Processes
- 9 Steps To Developing a Language Learning App
- A 1-Click Phone Data Transfer Method for Android to iPhone
- A Guide To 3D Automated Optical Inspection
- A Guide to Starting a Payment Processing Company
- A Tech Career: Is a B.Tech CSE the first step?
- Advanced Reporting Techniques for Selenium Test Results
- Advancing Efficiency with Power Monitoring and Quality Solutions
- AI Detectors: Safeguarding Authenticity in the Digital World
- All About Snapchat Premium
- Amazon is Planning to Launch a Smart Fridge
- API Testing and Why It’s Essential
- Apple Number 1 in Smartphone Sales for Q4 2021
- Are iPhone 15s Pushing Mobile Gaming Developers to New Levels?
- Avica Remote Desktop Review: Secure, Simple and Seamless
- Banjo-Kazooie to Arrive on Nintendo Switch Online
- Basis of EMI Licensing in UK
- Benefits of Automating IT Asset Discovery
- Benefits of Hiring an Outsource Firm
- Benefits Of Using Token Authentication For Your Security
- Best cases for Google Pixel 7
- Best file managers for Android: 10 proven options
- Best Free PDF Editor for College Students to Level Up Productivity
- Best Hard Drive Recovery Software: Wondershare Recoverit
- Best Methods to Protect Your Router from Viruses
- Best way to Transfer Data from iPhone to Samsung Galaxy Series
- Big Brother Era: Is Biometric Espionage a New Reality?
- Boost Office Productivity With These Easy Actions
- Boosting E-Commerce Sales through Accurate Email Address Lists
- Bridging the Knowledge Gap: How Retrieval-Augmented Generation Transforms AI Responses
- Business Applications and Benefits of Professional Outbound Calling
- Buy Real YouTube Subscribers: Buy Organic YouTube Subscribers USA
- Bypass FRP Lock on Xiaomi: A Comprehensive Tutorial
- C-Suite Insights: 5 Benefits of Software Outsourcing
- Can Short-Term Lenders Aid in Improving Your Credit Score
- Collection of Watermark Removal Tools: How to Get Rid of Watermarks Across Different Media
- Combating Climate Change With New Technologies
- Commercial Benefits of Using Solar Rooftop Panels for Businesses
- Common Mistakes To Avoid In Market Research
- Comparison between Germany and Singapore (Asia) VPS
- Condo Tech Revolution: How Apps Are Changing the Way We Live and Interact
- Could Artificial Intelligence Deliver Cheaper Car Insurance?
- CPUs and GPUs to Get Price Hikes in 2022
- Creating Websites is Not Just About Coding Anymore
- Crypto.com Loses $15M in Ethereum in Hack
- Cute and practical office helper: Akko Doraemon Macaron 3098B
- Cyberattack causes major disruption to UK medical helpline ‘120’
- Data Entry Virtual Assistants: Boosting Productivity
- Demystifying VPN: Exploring the Differences between Personal and Business VPNs
- Designing Effective Visualizations for SQL Reports
- Different Types of Microphones
- Digitizing High-Volume Document Workflows: Why File-Heavy Teams Need Better eSignature Infrastructure
- Discover the Benefits of Mobile apps for Entertainment
- Discover the Brilliance of the OPPO A79 5G Sunlight Display: A Visual Revolution for Mexican Smartphone Users
- DLP and Cybersecurity: Building a Comprehensive Defense Strategy
- Do I Need a VPN in the UAE?
- Do You Need an Instagram Follower Tracker?
- Download Windows Backup Software: Protect Your Data
- Effective payment methods for Google Ads: tips for choosing payment cards
- Elevate Your Server Management: Windows Servers + RDP
- Embarking on the Tech Journey: How Cloud-Based Solutions Propel Small Businesses
- Emotional Design: Creating Products That Forge Deeper User Connections
- Eneba Brings Down God of War PC’s Price to Just $37.76
- Enhancing Connectivity: Innovative Solutions for Today’s IT Challenges
- Essential Tech Items For Your New Condo
- Exploring Data Science with Python
- Field Service Management Software: Your Key to Streamlining Business Operations
- Financial Services You Can Find Online
- Flipbooks: A New Era in Digital Publishing
- Flutter Vs React Native For App Development: Which Is Better in 2022?
- Fortifying Your Digital Castle: The Power of ProGuard Security
- From Discovery to Protection: How DSPM Secures Your Data Lifecycle
- From Traditional Banking to Online: Savings Account Transformation
- Future Trends of Data Consulting
- Garmin Launches Fenix 7 Smartwatch Lineup
- Generate Dynamic QR Code To Have A Flexible Campaign
- Get Better at Blog Writing: 5 Essential Steps
- Give life to old memories with AI-powered photo restoration tools
- Guide to Creating Digital Marketing Strategy for Small Business
- Hadoop Backup Solutions and Large Data Pools
- Harmful SEO Links – How to Identify Them
- Headliners Media: Accelerating Growth Online for Startups, SMBs, and Growing Enterprises with PR, Content Marketing & SEO
- How AI Apps Are Ushering in a New Era for Product Photography
- How AI Can Help Financial Advisors Grow
- How AI is Transforming Retail with Voice Technology
- How AI Will Help Data Analytics
- How can ISO 9001 improve your business?
- How Data Fabric Can Improve Efficiency and Performance in the Workplace
- How Do I Find Cable Internet in My Area?
- How Does Website Designing Influence Search Engine Optimization?
- How Dynamic Case Assignment is Elevating Customer Service in the Travel Industry
- How IoT technology can make our house smart?
- How Keyless Entry Can Boost Your Home Security Efforts
- How Net Worth Calculators and Financial Apps Can Help You Achieve Your Financial Goals
- How Remote Developers are Reshaping the Software Industry?
- How Technology Can Help You Stay Focused at Work
- How to Browse Safely and Anonymously Online
- How to Choose the Best Google Ads Service Provider for Your Brand
- How to Collect and Track Payments like a Pro with Virtual Account API
- How to Complete Registration and Receive SMS without a SIM card
- How to Convert Your Digital COVID-19 Vaccination Card into a QR Code?
- How to Create a Successful SaaS SEO Strategy in 2022
- How to create a website design concept in 5 steps
- How to Create Amazing Graphics with Your Smartphone
- How To Create An Avatar With Picrew
- How to Create Training Videos with AI: A Step-by-Step Guide
- How to Develop Mobile Applications
- How to Draw a Circuit Diagram Easier With EdrawMax
- How to Encrypt Email?
- How to Enhance Safety When Sending Encrypted Online Message
- How to Find Best SEO Company in Hong Kong
- How to Find the Best Deals on AppHut?
- How To Get A Disposable Virtual Number For SMS Verification
- How to Hide Your Device’s Location
- How to Improve JPEG Image Quality: 5 Best Ways
- How to Limit Screen Time Easily on Android and iPhone with Parental Control App
- How to Move Files Between Cloud Storage – Easy & Free
- How to prevent entertainment apps from stealing your data and personal information
- How to Recover Data from Mac External Hard Drive
- How To Recover Deleted Files From an SD Card
- How to Recover Lost or Deleted Data on Windows 11?
- How to Recover RAID Data with RAID Recovery Software?
- How to Recover Vanished Video Files
- How to Repair Corrupt MKV Video Files?
- How to Repair Damaged PDF Files
- How To Start DevOps: A Complete Guide
- How to Transfer Data From iPhone to iPhone/Android Wirelessly
- How to Transfer Photos/Videos from iPhone to iPhone/Android Wirelessly
- How to Unlock iPhone without Passcode the Easy Way?
- How You Can Bring Historical Characters Back to Life with Face Swapping in Video Online
- How Your Smartphone Can Be Your Pocket-Sized Relaxation Tool
- HubSpot Content Hub vs. Traditional CMS: Which Is Better?
- Importance of Document Management Software to the Law Firm
- Important Areas to Include in a Tech Business
- Introduction to NLP Library: SpaCy Library in Python
- iPhone Data Transfer App – FoneTool Free Download & Reviews
- Is It a Good Idea to Buy a Refurbished iPhone?
- Is SQL going to be replaced in the Future?
- Lower Your Cell Phone Bill: Tips and Tricks
- Mastering Data Flow with ETL Processes
- Meeting Aerospace Electronics Demands with CNC Aluminum
- Microsoft Acquires Activision Blizzard for $68.7 Billion
- Mobile Application Development: Top Factors to Consider for Success
- Mr. Beast Tops Forbes’ ‘YouTube Content Creator’ List for 2021
- MuleSoft: A Comprehensive Guide to Integration Platform, Benefits and Use Cases
- Must Knows If You’re Getting Started with Workload Automation
- MyRecover – Windows Data Recovery Software
- Navigating the Cost of Restaurant Management Software Development: Market Forecast in 2024 and Key Considerations
- Navigating the UX Design Process from Research to Testing
- Navigating the Web Design Maze: A Small Business Owner’s Ultimate Guide
- Next-Gen Computers: Exploring the Latest Technological Advancements
- Optimizing Google Ads Payments: A Guide to Selecting the Right Payment Cards
- Picking Between Dedicated Servers and VPS at USA Datacenter
- PicWish: The Best AI Photo Enhancer and Editor
- Plesk Control Panel: Simplifying Web Hosting on VPS for Windows and Linux
- Preventing the 4 Major Multi-Cloud Security Challenges
- PropTech and Energy Management as Business Catalysts
- Pros and Cons of Multitasking While Working From Home
- Protect your AI Infrastructure with a DSPM Tool
- Proven Benefits of Hiring a Social Media Agency
- Python Array: What Is It?
- Python vs Java: Differences that Developers Should Know
- Quill Forms VS Gravity Forms
- Recover Deleted Files in Windows 10 With/Without Software
- Recruitment Efforts with the Top-Rated Free CRM
- Reliable VMware Backups and Rapid Restores
- Remote Control PC from iPhone with AnyViewer
- Restoring Windows to Previous Date [Windows 11/10]
- Revolutionizing Business Operations with Digital Forms
- Samsung Adopts AMD GPUs For Its Latest Mobile Devices
- Screen Recording Software Comparison: Finding the Right Solution for Your PC
- Secrets to Moving House Quickly Using Technology
- Secure File Hosting: How to Keep Your Data Protected in 2025
- Securing Online Communication with Microsoft Teams
- Securing Your SaaS Landscape: A Multi-Layered Defense Against AI Risks
- SEO Timeframes – Understanding the Factors that Influence Results
- Should Apartment Marketers Use Chatbots On Their Websites?
- Smart Heating and Cooling Systems
- Smart Living: How Condo Residents Can Benefit from Mobile Apps
- Smart Strategies to Boost Your SERP Rankings
- Social Surge: The Ultimate Guide to Buying Instagram Followers from Reputable Sites
- Solana Volume Bot: A New Force in Blockchain Trading
- Speed and Security: Why VPS is Essential for Hosting Large Files
- Speeding Up Response Times in the SOC with AI
- Splunk: The All-in-One Solution for Big Data Analytics
- Strategies for Increasing Employee Engagement with WFM Solutions
- Strengthening digital trust as the foundation for digital security
- Tailored Websites For Tech: Design Principles Every Msp Should Implement
- Take Control of Your VPS: Why Control Panels are an Indispensable Tool
- Takeaway Tips To Making The Most Of Your Collaboration With Android App Development Company
- Tech Savvy Tips for Better Health
- Tech That Changed Money Forever
- TED Talks That Anyone Working in List Open Ports on Linux Should Watch
- The 4 Features of Enterprise Secure File Sharing
- The Advantages Of Aluminium Extrusion Manufacturing In China
- The Anatomy of a Data Breach: From Attack Vectors to Remediation
- The Art of Anonymity: How Proxy VPNs Protect Your Digital Identity
- The Benefits of Data Mesh Technology for Businesses
- The Benefits of Outsourcing IT Services
- The Benefits Of Using An Updated Facility Management Software
- The Benefits of Using FamiSafe to Track Cell Phone Locations
- The Business Value of Good App Design
- The Critical Role of Electronic Logging Devices (ELDs) in Truck Accident Cases
- The Difference Between Conversion APIs and Pixels
- The Easy Fix for iPhone Error 4013 using the Best Software for Mac and PC
- The Evolution of Cyber Threats: How Data Detection and Response Keeps Pace
- The Future of AI Automation: Comparing GPT-4.5 API and Gemini 2.5 API for Workflow Optimization
- The Future of Automotive Technology: Impact on Insurance and Insurers
- The Future of Fax: Exploring Innovation in iPhone Apps
- The Game-Changing Role of Cloud-Based Data Storage Solutions
- The Great App Debate: Progressive Web Apps vs. Native Apps
- The Impact of Social Emotional Learning (SEL) in Schools: Fostering Emotional Intelligence and Well-being
- The Impact of Website Speed on User Experience and SEO
- The Importance of a Durable Solar Panel: Choosing Jackery for Long-lasting Power
- The Importance of Mobile-Responsive Design
- The Importance of Seed Keywords and Keyword Intent in Ecommerce SEO
- The Key Components of Internal Developer Platforms
- The Most Advanced Technologies Used By Online Businesses Nowadays To Protect And Store Sensitive Data
- The Most Promising Wearable Medical Devices to Invest In Today
- The Most Recommended Android App to Transfer Data Between Devices
- The Power of a Dedicated Server: A Software Developer’s Dream Machine
- The Power of UiPath_ Revolutionizing Automation
- The Quick and Easy Ways to Transfer Data from Samsung to the iPhone 15
- The Redmi K50 Series Could Rival iPhone’s Haptics Technology
- The Rise of Customisable iPads: Will Modular Design Be the Next Big Thing?
- The Rise of Smart Condos: How Technology Is Enhancing Resident Experience
- The Role of Custom Software Solutions in Transforming Cloud Storage
- The role of real-time monitoring software in reducing call center AHT
- The Role of Technology in Nobul’s Real Estate Approach
- The Top European Cloud Storage Solutions
- The Ultimate Guide to Creating Compelling Audiobooks with AI Voice Generator
- The Use of AI in Search Engine Optimization
- The UX Design Process – From Research to Testing
- Things to Check While Buying a Used iPhone
- Tips for a Smooth Website Migration: Moving Your Site to a New Host
- Tips for Creating a Data Loss Prevention Policy
- Tips for Using Digital Marketing Tools To Improve Your SEO
- Top 4 Ways to Fake GPS Location on iPhone Safely and Efficiently
- Top 5 Ecommerce SEO Strategies for Boosting Your Online Sales
- Top 6 Online AI Video Translator Review in 2023
- Top 6 Perks of Web Designing Services in Website Optimization
- Top 7 AI Apps to Boost Business and Productivity
- Top 7 iOS System Repair software in 2022 (Windows and Mac)
- Top API Protection Tools
- Top Off-Page SEO Trends That You Need To Check Out
- Top Online Bootcamps for UI/UX in 2022
- Top Software Testing Certifications for 2024
- Top Software Testing YT Channels for 2024
- Top Tools to Consider for Docker Development
- Top VPN Services for Enhanced Privacy and Security
- Top Way to Sync Google Photos to Google Drive
- True or Fake AI Data Repair Tool?
- Twill: The Best News Payment Platform for Businesses
- Types of Social Engineering Attacks in 2022
- Ultrasonic Flow Meter: What Is It? How Does It Work?
- Understanding IR Sensors: A Comprehensive Guide
- Understanding Search Algorithms Curated By Tech Enthusiasts
- Understanding Wildcard SSL certificates: What they are and how they work?
- Unlock the Full Potential of Office 365: Proven Tips and Tricks
- Unlocking the Potential of 3D Printing Services with the 3DEXPERIENCE Marketplace
- Unlocking Your GPS: Top Location Spoofer Apps for 2023
- Unveiling Plesk Web Pro Edition: Empowering Web Hosting Management
- Using OnlineSim for SMS Verification Codes
- Valuable Tips to Help You Select a Good Spy App
- Virbo vs Elai: AI Avatar Battle – Features, Pricing, and Performance
- Virtual Assistants in Improving Healthcare Workflow
- VoIP Caller ID and Other Features
- What are the Features of Pendo Alternatives?
- What Are the Key Features of Call Center Software?
- What Do You Need For IPTV Video Streaming?
- What Does CFS Mean On Instagram
- What innovations have recently taken place in the world of web hosting?
- What is a Gaming PR Agency and why do gaming businesses need them?
- What is a PAT, Aka Portable Appliance Tester?
- What Is a Smart Home and Why You Need to Invest in One?
- What Is a Subdomain and How Does It Impact SEO
- What is Antivirus Software?
- What is Content on Websites? Why You Need Moderation
- What is HR Technology and How It Can Help Your Company Achieve More
- What is MLOps and why is it important in today’s world of data-driven decision making
- What Is Tezos Blockchain Network – Should You Invest in It?
- What Skills are Required for C++ Engineer?
- What to Know About Contextualized Data
- What You Need to Know Before Choosing Your Website Host
- What’s new in Cloud Storage? A look at the Latest Offerings from Cloud Storage Providers
- Which Coffee Printer Suits me?
- Why 73% of Enterprises Choose Atlassian Consultants Like Evelon in 2025
- Why Accounting Software is Essential for Comprehensive Business Management in India
- Why an Enterprise Virtual Data Room Is Essential for Your Business
- Why can’t Retail Businesses Survive without Address Verification Services?
- Why Data and Call Center Analysis is Crucial for Your Customer Services
- Why do you need regression testing & how to successfully perform it?
- Why Every Agency Must Update About SEO Latest Techniques?
- Why Is Visual Design Important Everywhere?
- Why Listing on the Top Online Business Directory is a Must-Have for Your Business
- Why Negative Reviews Help Your Online Business
- Why Professional EV Charger Installation is Crucial for Safety and Longevity
- Why React Native is the Go-To Choice for Startup Mobile App Development
- Why Simulcast Is The Best Way To Expand Your Reach
- Why You Should Host Your Website on A Cloud Server and Not on an ordinary One
- Why You Should Use Pixlr to Optimize AI Artwork
- WIFI PTZ Battery + Solar Camera
- Windows Server Management: Tools and Techniques for Efficient Administration
- Winter-Proof Your Home: Your Essential Guide to Heating System Maintenance and Efficiency
- Your Ultimate Guide to GPS Tracking for Fleet Vehicles
- Youtube Mp3 Downloader Online For Mac
- YouTube’s Original Content Head Exec to Exit in March